Flow Control in Python
Contents
What are Control Flow statements?
We often come across situations in which we need to divert or change the usual sequential flow of execution. The flow control statements can be classified into Conditional Statements and Iteration Statements. The Conditional Statements selects a particular set of statements for execution depending upon a specified condition. While the Iteration Statements repeatedly executes a block of statements with respect to some condition. This article assumes that you have basic knowledge in programming languages like C. If you haven’t yet started with Python, please read the article Getting Started with Python.
if … elif … else … statements
The most popular conditional control statement is ‘if else’, so let’s see how it works in python.
if condition: statement1 statement2... elif condition: statement1 statement2... else: statement1 statement2... statementx
You might have already spotted the changes when compared to other programming languages. The changes improve the flexibility and ease of programming in python. All the conditional statements are always followed by a colon “:”. The codes under a block can be identified by the indentation. Thus maintaining proper indentation is critical in flow control statements. Let’s see an example.
x = int(input("Please Enter and an Integer : ")) if x > 0 : print ("The Number is Positive") elif x == 0 : print ("The Number is Zero") else : print ("The Number is Negative") print ("The End")
The above program checks whether a number is positive, negative or zero and the output is shown below.
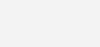
for loop
For is an iterative control statement, which repeatedly executes a set of statements depending up on some specified conditions.
Let’s see how it works
names=['ABC','BCD','EFG','FGH'] for i in range(0,4): print (names[i])
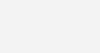
The range function can be used in many ways. As shown below..
- range(n) generates 0, 1, 2, 3 ….. n-1
- range(a,b) generates a, a+1, a+2 ….. b-1
- range(a,b,c) generates a, a+c, a+2c, ….
Example :
- range(10) generates 0, 1, 2, 3, 4, 5, 6, 7, 9.
- range(1,5) generates 1, 2, 3, 4.
- range(0,10,3) generates 0, 3, 6, 9.
break and continue Statements
Similar C programming, break statement is used to stop execution of the smallest enclosing loop, while the continue statement is used to skip the current iteration in a loop and continue with the next iteration. Let’s see it with a simple example.
for i in range(10): if (i==5): continue print(i) print('\n') for i in range(10): if (i==5): break print (i)
In the first loop when the value of ‘i’ is equal to 5 the current iteration is skipped and the loop continues execution from next iteration ie, values from 6 to 9. Whereas in the second loop when i becomes equal to 5 the break statement is executed and thus the execution of that loop is terminated as shown below.
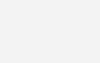
while loop
As in other programming languages the while loop is an entry control iterative looping statement. The difference lies in the fact that the inner statements are written with an indentation of one ‘tab’ space.  A simple program is illustrated here and the output also is shown.
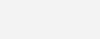