Interfacing LCD with 8051 using Keil C – AT89C51
Contents
Liquid Crystal Display (LCD) is very commonly used electronic display module and having a wide range of applications such as calculators, laptops, mobile phones etc. 16×2 character lcd display is very basic module which is commonly used in electronics devices and projects. It can display 2 lines of 16 characters. Each character is displayed using 5×7 or 5×10 pixel matrix.
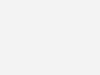
Interfacing 16×2 LCD with 8051 using Keil C is bit complex because there is no powerful libraries in Keil C. To solve this problem we have developed a LCD library which includes commonly used features, you just need to include our header file and use it. You can download the header file at the bottom of this article.
LCD can be interfaced with microcontroller in 4 Bit or 8 Bit mode. These differs in how data is send to LCD. In 8 bit mode to write a character, 8 bit ASCII data is send through the data lines D0 – D7 and data strobe is given through E of the LCD. LCD commands which are also 8 bit are written to LCD in similar way.
But 4 Bit Mode uses only 4 data lines D4 – D7. In this mode 8 bit character ASCII data and command data are divided into two parts and send sequentially through data lines. The idea of 4 bit communication is used save pins of microcontroller. 4 bit communication is a bit slower than 8 bit communication but this speed difference can be neglected since LCDs are slow speed devices. Thus 4 bit mode data transfer is most commonly used.
Library Functions in lcd.h
Lcd8_Init() & Lcd4_Init() : These function will initialize the LCD module which is connnected to pins defined by following bit addressable variables.
For 8 Bit Mode:
//LCD Module Connections sbit RS = P0^0; sbit EN = P0^1; sbit D0 = P2^0; sbit D1 = P2^1; sbit D2 = P2^2; sbit D3 = P2^3; sbit D4 = P2^4; sbit D5 = P2^5; sbit D6 = P2^6; sbit D7 = P2^7; //End LCD Module Connections
For 4 Bit Mode :
//LCD Module Connections sbit RS = P0^0; sbit EN = P0^1; sbit D4 = P2^4; sbit D5 = P2^5; sbit D6 = P2^6; sbit D7 = P2^7; //End LCD Module Connections
These connections must be defined for the proper working of the LCD library.
Lcd8_Clear() & Lcd4_Clear() :Â These functions will clear the LCD screen when interfaced with 8051 in 8 bit and 4 bit mode respectively.
Lcd8_Set_Cursor() & Lcd4_Set_Cursor() : These functions are used to set the cursor position on the lcd screen. By using this function we can change the position of character and string displayed by the following functions.
Lcd8_Write_Char() & Lcd4_Write_Char() : These functions are used to write a character to the LCD screen.
Lcd8_Write_String() & Lcd8_Write_String() : These functions are used to write a string or text to the LCD screen.
Lcd8_Shift_Left() & Lcd4_Shift_Left() : These functions are used to shift display left without changing the data in display RAM.
Lcd8_Shift_Right() & Lcd8_Shift_Right() : These functions are used to shift display right without changing the data in display RAM.
8 Bit Mode LCD Interfacing
Circuit Diagram
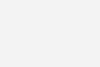
Keil C Code
#include<reg52.h> //including sfr registers for ports of the controller #include<lcd.h> // Can be download from bottom of this article //LCD Module Connections sbit RS = P0^0; sbit EN = P0^1; sbit D0 = P2^0; sbit D1 = P2^1; sbit D2 = P2^2; sbit D3 = P2^3; sbit D4 = P2^4; sbit D5 = P2^5; sbit D6 = P2^6; sbit D7 = P2^7; //End LCD Module Connections void Delay(int a) { int j; int i; for(i=0;i<a;i++) { for(j=0;j<100;j++) { } } } void main() { int i; Lcd8_Init(); while(1) { Lcd8_Set_Cursor(1,1); Lcd8_Write_String("electroSome LCD Hello World"); for(i=0;i<15;i++) { Delay(1000); Lcd8_Shift_Left(); } for(i=0;i<15;i++) { Delay(1000); Lcd8_Shift_Right(); } Lcd8_Clear(); Lcd8_Write_Char('e'); Lcd8_Write_Char('S'); Delay(3000); } }
4 Bit Mode LCD Interfacing
Circuit Diagram
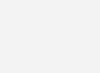
Keil C Code
#include<reg52.h> //including sfr registers for ports of the controller #include<lcd.h> //Can be download from bottom of this article //LCD Module Connections sbit RS = P0^0; sbit EN = P0^1; sbit D4 = P2^4; sbit D5 = P2^5; sbit D6 = P2^6; sbit D7 = P2^7; //End LCD Module Connections void Delay(int a) { int j; int i; for(i=0;i<a;i++) { for(j=0;j<100;j++) { } } } void main() { int i; Lcd4_Init(); while(1) { Lcd4_Set_Cursor(1,1); Lcd4_Write_String("electroSome LCD Hello World"); for(i=0;i<15;i++) { Delay(1000); Lcd4_Shift_Left(); } for(i=0;i<15;i++) { Delay(1000); Lcd4_Shift_Right(); } Lcd4_Clear(); Lcd4_Set_Cursor(2,1); Lcd4_Write_Char('e'); Lcd4_Write_Char('S'); Delay(2000); } }
How to use the Header File ?
Copy and Paste the header file lcd.h to your project folder. Then add lcd.h to your source group.
1. Right Click on Source Group
2. Click on “Add Files to ‘Group Source Group 1’
3. Select lcd.h and Click Add
Optimize Code for more Efficiency
You already seen that by using our header file lcd.h you can connect LCD to any of the output pins of the microcontroller. For this more coding is required in the header file which makes the generated hex file less efficient and large in size. You can solve this problem by making some changes in the header file according to your hardware connections. For eg : in the above sample programs I have used port P2 for sending data and command. Just replace the functions Lcd4_Port(data) and Lcd8_Port(data) with P2 = data.
Download Here
You can download header file, keil c files, proteus files etc here…
how to write on lcd it just glows but i want to write on lcd
c
s
Please I have been trying to add a delete function that deletes a character when initiated please help
lcd.h header file should be save in new text of same project.
There will be two files in your project case Text1.c and lcd.h
I want to display a variable. I declared it under int. What do I do now?
there is some mistake in this cmd please change it sir
you didnt initilalize the basic functions like void lcd_cmd() void lcd_data() void lcd_init() after calling each function with proper statement it will work
connect a resistor array with lcd connection thanku
try adding prototype in your program.
Hi Sir,
Everytime I build and compile my program in keil, warning C206 always pops up and says:
LCD.C(37): warning C206: ‘Lcd8_init’: missing function-prototype
the Lcd8_init is properly declared and defined in your header file “lcd.h” and yet this warning is displayed. What might be the solution for this?
By the way sir, thanks for the examples. I learned a lot from you. The program is working properly both in simulation and hardware.
Thanks,
Cris
C function names are case sensitive.
Sorry, can you please elaborate ?
hi sir how can I fix this error
can you tell me the program for lcd interfacing to display full name on lcd using pointer?
http://www.keil.com/support/man/docs/c51/c51_c267.htm
Just try adding a prototype.
Make sure the the LCD connections are correct.
it does,nt work..plz help??
nnnn.c(34): error C267: ‘Lcd8_Write_String’: requires ANSI-style prototype
Target not created.
Build Time Elapsed: 00:00:00
hi am tring to interface the lcd with 8051 microcontoller. it is showing garbage value can someone please help??
Just try downloading and simulating our example files.
i m facing the same problem.. please help me out.. whats the solution?
Try adjusting it practically or calculate the cycles required.
It will work. Please post more details.
any please help me to get 1 second delay
please guys any one help me to get 1 second delay
in this program .i cont able to find error
#include
sbit rs=P1^0;
sbit rw=P1^1;
sbit en=P1^2;
void lcd_cmd(unsigned char a);
void lcd_data(unsigned char b);
void lcd_ini();
void delaye();
void delay();
void main()
{
char d[]=”abcdefghijklmanopqrstuvwxyz”,i;
lcd_ini();
for(i=0;d[i]!=”;i++)
lcd_data(d[i]);
}
void lcd_ini()
{
lcd_cmd(0x38);
lcd_cmd(0x06);
lcd_cmd(0x0F);
}
void lcd_cmd(unsigned char a)
{
rs=0;
rw=0;
en=1;
P2=a;
delaye();
en=0;
}
void lcd_data(unsigned char b)
{
rs=1;
rw=0;
en=1;
P2=b;
delay();
en=0;
}
void delaye()
{
{
char c;
for(c=1;c<=1;c++)
{
TMOD=0x02;
TH0=0xB8;
TL0=0x63;
TR0=1;
while(!TF0);
TR0=0;
TF0=0;
}
}
} void delay() //1 second delay
{
{
char c;
for(c=1;c<=20;c++)
{
TMOD=0x02; //50 ms 50*20=1000ms=1 second
TH0=0X4B;
TL0=0xFF;
TR0=1;
while(!TF0);
TR0=0;
TF0=0;
}
}
}
I compile the code but it doesnt work in the simulation. The lcd doesnt display anything. Please help
Try using sprintf()
You can open the lcd.h header file for that.
how can i display an integer on LCD in 4 bit mode whose value is changing if i press a switch
sir i wish to to see the c coding for lcd header file.
Include the file lcd.h to your project.
Lcd8_set_cursor.. missing function prototype.. how could i get rid of it?
thanks sir,,,for your support…
Do right click on micro controller, where the last option is add/remove source file. Select the hex file of your code. Make sure that in your program you use port P2 for output and connect your remaining lines of lcd (ie D0-D7) to respective ports in lcd.
Hi, I corrected the header file. Please download again and check.
the string is not displaying in 2nd row of LCD in 4 bit mode please check it sir..
Hi, I am working on this project (Temperature Indicator using AT89C52) currently and I couldn’t find a 16×1 LCD. So got a 16×2 LCD display. Is there any workaround to get this project working using the 16×2 LCD ?
And what hardware will be required to interface the LCD with the microcontroller(AT89C52) ?
Much Thanks in advance!
It is already shown in above example.. using left and right shift functions..
please tell me how to scroll string on lcd?
It is 100% working..
Thanks
this code is not work
Just convert it to string and print it…
You can convert a single digit number to character by adding 48;
‘1’ = 1 + 48
‘2’ = 2 + 48
How do I print value of any variable that I have us in my program. .?
it doesnt work beacause p0 is open drain , use pull up resistors ! watch how to use P0 on google
Please give me more details about the error..
I have been trying my best to make an LCD unit, which I’m using as part of an MCU 8051 IDE project.
Still not able to get it to work. There is always some error or the other. It will sometimes say syntax error for tokens such as “=”, and when I change the header files and some settings, it gives me an error that file .cdb was not found.
This is very distressing as I have literally tried almost every combination of header files, sdcc versions, and what not.
Copy the file lcd.h to your project folder and add it to Source File Group.
I really don know how to solve it. Plz help me
I really don know how to solve it. Plz help me
Ya I did that and it worked 🙂 Thanks 🙂
convert variables to character and print it.
How to print variables?
Sorry, we aren’t providing any software. You can buy it from Labcenter Electronics.
Yes , rite … i was able have it display as well . thanks . Can i please get the latest version of proteus 8.1 or 2 .
Hello,
The Proteus simulation for both 4 bit and 8 bit are working fine for me..
hi i tried the same code which is present in the downloaded zip file .
I have simulated the given circuit but when i run the simulation nothing is being displayed on the LCD .
Please help me .
thanks
It is Proteus ISIS
Download an try my file..
Which software is this sir?
Sir
I want lcd.c file in which all the functions have been defined so that i can look how functions work.
a is the string to be displayed
eg : Lcd8_Write_String(“Paramesh”);
sir i have doubt in
void Lcd8_Write_String(char *a)
{
int i;
for(i=0;a[i]!=”;i++)
Lcd8_Write_Char(a[i]);
}
in what is the stored in *a?
what is the stored in a?
Look at the header file lcd.h
can i get d complete details about d ports used in tis?
I compile the code but it doesnt work in the simulation. The lcd doesnt display anything. Please help
May i know how many addressable unit are there in the above LCD panel? and what are they??? Please let me know soon..
compiling lcd.c…
lcd.c(2): warning C318: can’t open file ‘lcd.h’
lcd.c(30): warning C206: ‘Lcd8_Init’: missing function-prototype
lcd.c(33): warning C206: ‘Lcd8_Set_Cursor’: missing function-prototype
lcd.c(33): error C267: ‘Lcd8_Set_Cursor’: requires ANSI-style prototype
lcd.c – 1 Error(s), 3 Warning(s).
suicide? WHY NOT!
die? YES!
die!
kill yourself!
kill Yourself
Kill yourself
kill yourself
diE
dIe
Die
die
your life is worthless
your life is meaningless
Hi my program is working in keil but when I run the circuit simulation LCD stays blank.
what would be the problem?
what is meant by these warnings
Build target ‘Target 1’
warning l1;unresolved external symbol
symbol:?c_startup
module: start.obj(?c_startup)
warning l2; reference made to unresolved external
symbol:?c_startup
module: start.obj(?c_startup)
address;080AH
Hey I want to display a variable value on LCD.. I am using 4 bit pattern.. I have the variable g (integer type) and I want to display the value of g on LCD as in
Lcd4_Write_Char(‘value of g’); Please help!!
It is working fine for me..
Hi my program is working in keil but when I run the circuit simulation the circuit stays blank !
There is a download link at the end of the article above…
Use the above download link..
whwre is the download link for downloading lcd.h headrefile??? i couldn’t find..
how to download lcd.h header file??
very Interesting.
You can use a series of delays….
sir,
can you please suggest me what actual value we have to take to provide 1 hour delay for the stepper motor
Just use the above header file………
i want to run a program of lcd interfacing with 8051 and display the reading of the meter in our lcd.
how to do so?
Try adjusting the contrast of the LCD using the preset… also check the connections of Enable and R/W pins of LCD..,,,
Try adjusting the contrast of the LCD using the preset… also check the connections of Enable and R/W pins of LCD..
I too hav the same problem now bkash. Help me, if you found solution to yours. And Ligo, there is no electrical problem. Its something else. But can’t find it. Please do help me,
Header file is present in the above download..
where is header file
When you assign a character to a variable.. you will get its ASCII value.. ….. to get its actual value of the digit you should convert it by subtracting 48.. try this link
http://www.asciitable.com/
i tried that way tooo but i can’t get the way to print value of the character variable to which answer is assigned.. what can i do?
Then you don’t need to read data from lcd… We can do it simply by storing that values in some variables.. before writing to LCD…
for example my code is like this,if the user presses ‘=’ key,then
void equalto()
{
if (variable2== operator) //*conditions will be written for all operators in nested if *//
{
variable4=variable1(/*here comes any operator/*variable2)variable3;
lcd8_write_char(value of variable4);
}}
the above fn will be called.
.. now how can i get the value of variable4 printed on lcd screen…
hope you are not confused and you got my logic..
my code is like this,if the user presses ‘=’ key,then
void equalto()
{
if (variable2== operator) //*conditions will be written for all operators in nested if *//
{
variable4=variable1(/*here comes any operator/*variable2)variable3;
lcd8_write_char(value of variable4);
}}
the above fn will be called.
.. now how can i get the value of variable4 printed on lcd screen…
hope you are not confused and you got my logic..
i’m planning the same also by using pointers..
to make a simple calculator , first user inputs digits along with operators in this format:
12*13
i want to read those operands and operators from screen and assign it to 3 variables, then proceed my calculation,…. is there any possibility??
Why do you want to read data from LCD screen???
There is an option in the LCD controller to read it… by using R/W pin of LCD….
Convert the variable to corresponding ASCII character… then simply display it…
Where is #define lcd p0?????
Can you please elaborate?
sir i cant undustand the function in lcd program that #define lcd p0
pls help me giving enough infomation
how can we make display the value of a variable here?
can we use ansi c command “%d”?????
can you please tell me how can we read a character from lcd screen ]
Copy the header file ‘lcd.h’ to your project directory and add the header file to your source group..
. Right Click on Source Group
2. Click on “Add Files to ‘Group Source Group 1′
3. Select lcd.h and Click Add
is der any video for adding header file??
following warnings r der…although i added lcd.c
Build target ‘Target 1’
compiling lcdsom.c…
lcdsom.c(2): warning C318: can’t open file ‘lcd.h’
lcdsom.c(30): warning C206: ‘Lcd8_Init’: missing function-prototype
lcdsom.c(33): warning C206: ‘Lcd8_Set_Cursor’: missing function-prototype
lcdsom.c(33): error C267: ‘Lcd8_Set_Cursor’: requires ANSI-style prototype
Target not created
We will explain it in detail in coming articles… Read the datasheet of LCD controller HD44780 used with this LCD Module..
i need explanation for every commands….if u can,pls mail me the algorithm for the 8 bit version…
[email protected]
Warnings will not stop your program from compiling..
What is the error?
Try the program files in the above zif file (download)…
I am unable to compile your program in uvision 4…. showing 6 warnings… please help.. unable to open the header file also.. shows an error for that….
in the function definition void Delay(int a), a is an argument of the function Delay(). Which means when we call the function delay, eg: Delay(1000), the argument a will be equal to 1000, a = 1000
sir, i have one doubt. in the fallowing code what is the value of ‘a’. I mean which is not assign to any value.
void Delay(int a)
if this ‘a’ is an accumulator, what is the value?
Thanks in advance.
Try adjusting the contrast of the LCD.
Make sure that the microcontroller program is running fine by blinking a LED.
Make sure that all pins are properly connected to LCD..
i have made a cirutial arrangement to displya message using keil …the code runs properly in proteus and the message is also diplayed well..but when i tried the same connection in hardware…nothing is showin up …only the black box are apperaing in the lcd..plz do help me with this matter..
It is not that much easy as your question. You require hardware knowledge and should write functions as required. Save these functions in a header file. Then you can include that header file.. when you require that functions…
teach me process of creating different libraries
Extract the above zif file.. Header file is in the Header File folder..
Unable to download that header file..please check
You can download entire project file from the above download link. .it includes lcd.h header file..
i can’t find header file? lcd.h