Interfacing GPS with Arduino
Contents
GPS stands for Global Positioning System. GPS module find the location (latitude, longitude, altitude) by comparing the time taken for receiving signals from satellites in its vicinity. GPS offers a great accuracy and also provides other data besides position coordinates.
Components Required
- Arduino Uno
- GPS
- Breadboard
- Connecting Wires
Hardware
Circuit Diagram
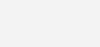
Connections
- Connect TX pin of GPS Module to pin number 4Â of Arduino Uno.
- Connect RX pin of GPS Module to pin number 3 of Arduino Uno.
- Connect Red wire (5V pin) of GPS module to 5V pin of Arduino Uno.
- Connect Black wire (GND pin) of GPS Module to GND pin of Arduino Uno.
Software
#include <TinyGPS++.h> #include <SoftwareSerial.h> SoftwareSerial GPS_SoftSerial(4, 3); TinyGPSPlus gps; volatile float minutes, seconds; volatile int degree, secs, mins; void setup() { Serial.begin(9600); GPS_SoftSerial.begin(9600); } void loop() { smartDelay(1000); unsigned long start; double lat_val, lng_val, alt_m_val; bool loc_valid, alt_valid; lat_val = gps.location.lat(); loc_valid = gps.location.isValid(); lng_val = gps.location.lng(); alt_m_val = gps.altitude.meters(); alt_valid = gps.altitude.isValid(); if (!loc_valid) { Serial.print("Latitude : "); Serial.println("*****"); Serial.print("Longitude : "); Serial.println("*****"); delay(4000); } else { Serial.println("GPS READING: "); DegMinSec(lat_val); Serial.print("Latitude in Decimal Degrees : "); Serial.println(lat_val, 6); DegMinSec(lng_val); Serial.print("Longitude in Decimal Degrees : "); Serial.println(lng_val, 6); delay(4000); } } static void smartDelay(unsigned long ms) { unsigned long start = millis(); do { while (GPS_SoftSerial.available()) gps.encode(GPS_SoftSerial.read()); } while (millis() - start < ms); } void DegMinSec( double tot_val) { degree = (int)tot_val; minutes = tot_val - degree; seconds = 60 * minutes; minutes = (int)seconds; mins = (int)minutes; seconds = seconds - minutes; seconds = 60 * seconds; secs = (int)seconds; }
Code Explanation
Include the “TinyGPS++.h” header file to include libraries for the communication with the GPS module. Create an object of the class SoftwareSerial and create an object named gps of the class TinyGPSPlus.
#include <TinyGPS++.h> #include <SoftwareSerial.h> SoftwareSerial GPS_SoftSerial(4, 3); TinyGPSPlus gps;
Initialize the variables with volatile float and volatile int type.
volatile float minutes, seconds; volatile int degree, secs, mins;
Put your setup or configuration code in the setup function, it will run only once during the startup. Define the baudrate as 9600 for serial communication and for GPS software serial communication.
void setup() { Serial.begin(9600); GPS_SoftSerial.begin(9600); }
Put your main code in void loop() function to run repeatedly. Get the latitude and longitude information from GPS and checks whether it is valid information or not.
void loop() { smartDelay(1000); unsigned long start; double lat_val, lng_val, alt_m_val; bool loc_valid, alt_valid; lat_val = gps.location.lat(); /* Get latitude data */ loc_valid = gps.location.isValid(); /* Check if valid location data is available */ lng_val = gps.location.lng(); /* Get longtitude data */ alt_m_val = gps.altitude.meters(); /* Get altitude data in meters */ alt_valid = gps.altitude.isValid(); /* Check if valid altitude data is available */
Checks for valid location data and displays “*****” on serial monitor in case of invalid location data reception.
if (!loc_valid) { Serial.print("Latitude : "); Serial.println("*****"); Serial.print("Longitude : "); Serial.println("*****"); delay(4000); }
Displays “GPS READING” message on serial monitor in case of valid location data reception. Convert the decimal degree value into degrees, minutes, seconds form and displays the location information on serial monitor in latitude and longitude.
else { Serial.println("GPS READING: "); DegMinSec(lat_val); Serial.print("Latitude in Decimal Degrees : "); Serial.println(lat_val, 6); DegMinSec(lng_val); Serial.print("Longitude in Decimal Degrees : "); Serial.println(lng_val, 6); delay(4000); } }
This loop provides sufficient delay to read the location data. Encode data read from GPS while data is available on serial port. Encode basically is used to parse the string received by the GPS and to store it in a buffer so that information can be extracted from it.
static void smartDelay(unsigned long ms) { unsigned long start = millis(); do { while (GPS_SoftSerial.available()) gps.encode(GPS_SoftSerial.read()); } while (millis() - start < ms); }
Convert data in decimal degrees into degrees, minutes, seconds form.
void DegMinSec( double tot_val) { degree = (int)tot_val; minutes = tot_val - degree; seconds = 60 * minutes; minutes = (int)seconds; mins = (int)minutes; seconds = seconds - minutes; seconds = 60 * seconds; secs = (int)seconds; }
Practical Implementation
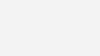
Working
In this project we aims to find out the coordinates of a place using GPS. Here we are using GPS module and Arduino Uno. GPS module finds the location (latitude, longitude) from the signals received from the satellite. Arduino reads information from the GPS module via serial communication (UART).
Video
Download
Click on the below link to download the Library file for GPS and include this library into Arduino IDE.
i have connected connections well as shown its showing an invaild data. even i have verifying the output in an openspace