Interfacing Stepper Motor with PIC Microcontroller
Contents
Introduction
A Stepper Motor is a brushless, synchronous DC electric motor, which divides the full rotation into a number of equal steps. It finds great application in field of microcontrollers such as robotics. Please refer the article Stepper Motor or Step Motor for detailed information about working of stepper motor, types and modes of operation. Unipolar Motor is the most popular stepper motor among electronics hobbyist because of its ease of operation and availability. Here I explaining the working of Unipolar and Bipolar Stepper Motor with PIC 16F877A Microcontroller.
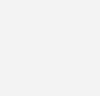
Stepper Motor can be easily interfaced with PIC Microcontroller by using readymade ICs such as L293D or ULN2003. As I said in the article Stepper Motor or Step Motor, we have three different types of stepping modes for unipolar stepper motor.
Note: 1 – Represents Supply Voltage and 0 – Represents Ground
Wave Drive
In this mode only one stator electromagnet is energised at a time. It has the same number of steps as the full step drive but the torque is significantly less. It is rarely used. It can be used where power consumption is more important than torque.
Wave Drive Stepping Sequence |
||||
---|---|---|---|---|
Step | A | B | C | D |
1 | 1 | 0 | 0 | 0 |
2 | 0 | 1 | 0 | 0 |
3 | 0 | 0 | 1 | 0 |
4 | 0 | 0 | 0 | 1 |
Full Drive
In this mode two stator electromagnets are energised at a time. It is the usual method used for driving and the motor will run at its full torque in this mode of driving.
Full Drive Stepping Sequence |
||||
---|---|---|---|---|
Step | A | B | C | D |
1 | 1 | 1 | 0 | 0 |
2 | 0 | 1 | 1 | 0 |
3 | 0 | 0 | 1 | 1 |
4 | 1 | 0 | 0 | 1 |
Half Drive
In this stepping mode, alternatively one and two phases are energised. This mode is commonly used to increase the angular resolution of the motor but the torque is less approximately 70% at its half step position (when only a single phase is on). We can see that the angular resolution doubles in Half Drive Mode.
Half Drive Stepping Sequence |
||||
---|---|---|---|---|
Step | A | B | C | D |
1 | 1 | 0 | 0 | 0 |
2 | 1 | 1 | 0 | 0 |
3 | 0 | 1 | 0 | 0 |
4 | 0 | 1 | 1 | 0 |
5 | 0 | 0 | 1 | 0 |
6 | 0 | 0 | 1 | 1 |
7 | 0 | 0 | 0 | 1 |
8 | 1 | 0 | 0 | 1 |
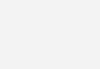
Driving Bipolar Motor
Bipolar motors are simpler in construction as it contains two coil and no centre tap. Being simple, driving is little complex compared to unipolar motors. To reverse the magnetic polarity of stator windings, current through it must be reversed. For this we should use H-Bridge. Here I using L293d, H-Bridge Motor Driver for that. We can distinguish bipolar motors from unipolar motors by measuring the coil resistance. In bipolar motors we can find two wires with equal  resistance.
Bipolar Motor Stepping Sequence |
||||
---|---|---|---|---|
Step | A | B | C | D |
1 | 1 | 0 | 0 | 0 |
2 | 0 | 0 | 1 | 0 |
3 | 0 | 1 | 0 | 0 |
4 | 0 | 0 | 0 | 1 |
Interfacing Unipolar Stepper Motor with PIC Microcontroller
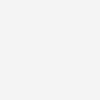
L293D and L293 are dual H-bridge motor drivers. The L293D can provide bidirectional drive currents of up to 600-mA at voltages from 4.5 V to 36 V while L293 can provide up to 1A at same voltages. Both ICs are designed to drive inductive loads such as dc motors, bipolar stepping motors, relays and solenoids as well as other high-current or high-voltage loads in positive-supply applications. All inputs of these ICs are TTL compatible and output clamp diodes for inductive transient suppression are also provided internally. These diodes protect our circuit from the Back EMF of DC Motor. In both ICs drivers are enabled in pairs, with drivers 1 and 2 are enabled by a high input to 1,2EN and drivers 3 and 4 are enabled by a high input to 3,4EN. When drivers are enabled, their outputs will be active and in phase with their inputs. When drivers are disabled, their outputs will be off and will be in the high-impedance state.
ULN2003 is a monolithic high current and high voltage Darlington transistor arrays. ULN2003 consists of seven NPN Darlington Transistor pairs that have high-voltage outputs with common-cathode clamp diode for switching inductive loads. The collector-current rating of a single darlington pair can be up to 500mA. We can connect Darlington Transistor pairs in parallel if higher current capability is needed..
Interfacing using L293D
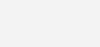
Note:Â VDD and VSS of the pic microcontroller is not shown in the circuit diagram. VDD should be connected to +5V and VSS to GND.
PORTB of PIC Microcontroller is used for controlling the stepper motor.
Interfacing using ULN2003
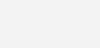
Note:Â VDD and VSS of the pic microcontroller is not shown in the circuit diagram. VDD should be connected to +5V and VSS to GND.
MikroC Programming
Since same pins of PIC Microcontroller is used for controlling Stepper Motor in both circuits, we can implement Wave, Full and Half Drives in the same circuit just by changing the program.
MikroC Code for Wave Drive
void main() { CMCON = 0x07; // To turn off comparators ADCON1 = 0x06; // To turn off analog to digital converters TRISB = 0; // PORT B as output port PORTB = 0x0F; do { PORTB = 0b00000001; Delay_ms(500); PORTB = 0b00000010; Delay_ms(500); PORTB = 0b00000100; Delay_ms(500); PORTB = 0b00001000; Delay_ms(500); }while(1); }
MikroC Code for Full Drive
void main() { CMCON = 0x07; // To turn off comparators ADCON1 = 0x06; // To turn off analog to digital converters TRISB = 0; // PORT B as output port PORTB = 0x0F; do { PORTB = 0b00000011; Delay_ms(500); PORTB = 0b00000110; Delay_ms(500); PORTB = 0b00001100; Delay_ms(500); PORTB = 0b00001001; Delay_ms(500); }while(1); }
MikroC Code for Half Drive
void main() { CMCON = 0x07; // To turn off comparators ADCON1 = 0x06; // To turn off analog to digital converters TRISB = 0; // PORT B as output port PORTB = 0x0F; do { PORTB = 0b00000001; Delay_ms(500); PORTB = 0b00000011; Delay_ms(500); PORTB = 0b00000010; Delay_ms(500); PORTB = 0b00000110; Delay_ms(500); PORTB = 0b00000100; Delay_ms(500); PORTB = 0b00001100; Delay_ms(500); PORTB = 0b00001000; Delay_ms(500); PORTB = 0b00001001; Delay_ms(500); }while(1); }
You can download MikroC Source Code, Proteus files etc here….
Interfacing Bipolar Stepper Motor with PIC Microcontroller
Bipolar Stepper Motor can be easily interfaced with PIC Microcontroller using L293D.
Circuit Diagram
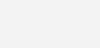
Note:Â VDD and VSS of the pic microcontroller is not shown in the circuit diagram. VDD should be connected to +5V and VSS to GND.
MikroC Code
void main() { CMCON = 0x07; // To turn off comparators ADCON1 = 0x06; // To turn off analog to digital converters TRISB = 0; // PORT B as output port PORTB = 0x0F; do { PORTB = 0b00000001; Delay_ms(500); PORTB = 0b00000100; Delay_ms(500); PORTB = 0b00000010; Delay_ms(500); PORTB = 0b00001000; Delay_ms(500); }while(1); }
Download Here
You can download MikroC Source Code, Proteus files etc here…
Hi Ligo George …
Please check this code ,
I have interfaced 2 amps (1.8 deg ) Stepper Motor to pic16f877a pic controller. Am unsign L298N Stepper motor driver .
#define A1 RC0 // OUTPUT motor
#define A2 RC1 // OUTPUT
#define B1 RC2 // OUTPUT
#define B2 RC3 // OUTPUT
#define pulse RD0 // INPUT pulse sensor
#define led RD2 // OUTPUT power on
#define DETECT RB3 // OUTPUT MAGNETIC SENSOR
while(1)
{
state = DETECT;
if(pulse && state == 1)
{
led = 1;
for(i=0; i<50; i++)
{
motor_full();
}
}
if(pulse == 0)
{
motor_off();
led = 0;
}
if(state == 0)
{
i++;
motor_full(); // come back to home position
}
}
}
void motor_full()
{
A1 =1; B1= 0; A2 =0; B2= 1;
__delay_ms(1);
A1 =0; B1= 0; A2 =0; B2= 1;
__delay_ms(1);
A1 =0; B1= 0; A2 =1; B2= 1;
__delay_ms(1);
A1 =0; B1= 0; A2 =1; B2= 0;
__delay_ms(1);
A1 =0; B1= 1; A2 =1; B2= 0;
__delay_ms(1);
A1 =0; B1= 1; A2 =0; B2= 0;
__delay_ms(1);
A1 =1; B1= 1; A2 =0; B2=0;
__delay_ms(1);
A1 =1; B1= 0; A2 =0; B2= 0;
__delay_ms(1);
}
void motor_off()
{
PORTCbits.RC0 = 0;
PORTCbits.RC1 = 0;
PORTCbits.RC2 = 0;
PORTCbits.RC3 = 0;
A1 =0; B1= 0; A2 =0; B2= 0;
}
It depends on the minimum stepping angle of your motor. What is minium step ?
Hi.. Ligo George
I want to rotate my stepper motor for 1.8 degree. For that what process i should follow. If i run this above program its working well and good but the rotation is about 45 degree for every cycle.
Sorry, the above article is not about Atmega. Please use our forums ( https://electrosome.com/forums ) for asking doubts outside the scope of above article.
HI…Ligo geroge
. I want to rotate unipolar stepper motor with atmega16a
and i configure it with following code
#include
#include
int main()
{
DDRA = 0xFF; //All pins of PORTB as output
PORTA = 0x00; //Initially all pins as output high
while(1)
{
PORTA = 0x01; //0001
_delay_ms(10);
PORTA = 0x04; //0100
_delay_ms(10);
PORTA = 0x02; //0010
_delay_ms(10);
PORTA = 0x08; //1000
_delay_ms(10);
}
return 0;
}
and common terminal connected to vcc
its not rotating properly
will you suggest me any solution
Thanks in advances
You can control the speed by adjusting the delay in each step and reading the potentiometer using adc.
You can calculate depending on the number of steps on that stepper motor.
You can use for loop for that.
sorry it is stepper motor
I am Mark, I need a code in MikroC to control the speed of my steeper motor using a potentiometer when the switch is on/ high. Thank you
Ligo i want to make 5 steps in one revolution…
Pls provide me code for mplab x…
Its urgent..
hello
I want to vary the speed using a potentiometer and I want to display this speed on an LCD , how to calculate this rate !!
Hello Ligo, please upload the program to stop the motor after rotating 50 steps (using 1.8 degree step. 1.8 x 50 = 90 degree )
and need to rotate back to 0 degree position after a few minute delay
NO.
MPLAB is an IDE only. MikroC is a compiler + ide.
For MPLAB you have to use Hi-Tech C, XC8 or XC18 etc..
Hello Ligo,is Micro C code going to work with MPLAB ?
Same as above.
how to interface two stepper motor with the pic 16F877
Thx Ligo
Sorry, I haven’t any experiences with Mobil Robot.
Ligo ,what do you think about stepper motor is it suitable for mobil robot?
You can use Geared DC Motor..
Sorry I haven’t any codes for that…. Read our DC Motor interfacing tutorial..
Hi .1st thx.I need to construct a mobol robot ,so wich kind of motor should i use to control it for forward ;backward;right and left?
Could u give me the code plz?
hello, my question… i follow this circuit….. my project i use 2 ldr as input at portA… but motor cannot move. why?? please reply or send to me the program. email :[email protected]… Urgent!!!
You needn’t use PWMs as you are using Stepper Motor….
You can adjust speed by changing the delays between steps
I want four steppers to be controlled from a single PIC16 or ATMega16. I want o have speed control (i.e the steps and the direction to be controlled by the user via a serial hyper -terminal). In that case do I need to use PWMs ?
How many PWMs are there on PIC16F877a ? Would I be able to run four motrs witha single MCU chip and L293D ?
0x indicates hex code…
0b indicates binary…
0 = 0000 in binary
F = 1111 in binary..
there for .. 0x0f in hex equal to 00001111 in binary..
0x0f = 0b00001111
What does 0x0f mean?I am newbie to mikro c.
can you please give me a sample program for five degree freedom robotic arm using servo motor? we are using mikroc in 16f84a as our pic and we are having a hard time solving it. thanks! 😀