Interfacing LCD with Atmega32 Microcontroller using Atmel Studio
Contents
As we all know LCD (Liquid Crystal Display) is an electronic display which is commonly used nowadays in applications such as calculators, laptops, tablets, mobile phones etc. 16×2 character LCD module is a very basic module which is commonly used by electronic hobbyists and is used in many electronic devices and project. It can display 2 lines of 16 character and each character is displayed using 5×7 or 5×10 pixel matrix.
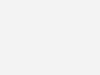
Interfacing 16×2 LCD with Atmega32 Atmel AVR Microcontroller using Atmel Studio is bit complex as there is no built in libraries. To solve this difficulty we developed a LCD library which includes the commonly used features. Just include our header file and enjoy. You can download the header file from the bottom of this article.
16×2 LCD can be interfaced with a microcontroller in 8 Bit or 4 Bit mode. These differs in how data and commands are send to LCD. In 8 Bit mode character data (as 8 bit ASCII) and LCD command are sent through the data lines D0 to D7. That is 8 bit data is send at a time and data strobe is given through E of the LCD.
But 4 Bit mode uses only 4 data lines D4 to D7. In this 8 bit data is divided into two parts and are sent sequentially through the data lines. The idea of 4 bit communication is introduced to save pins of microcontroller. 4 bit communication is bit slower than 8 bit but this speed difference has no significance as LCDs are slow speed devices. Thus 4 bit mode data transfer is most commonly used.
Function in lcd.h
Lcd8_Init() & Lcd4_Init() :Â These functions will initialize the 16×2 LCD module connected to the microcontroller pins defined by the following constants.
For 8 Bit Mode :
#define D0 eS_PORTD0 #define D1 eS_PORTD1 #define D2 eS_PORTD2 #define D3 eS_PORTD3 #define D4 eS_PORTD4 #define D5 eS_PORTD5 #define D6 eS_PORTD6 #define D7 eS_PORTD7 #define RS eS_PORTC6 #define EN eS_PORTC7
For 4 Bit Mode :
#define D4 eS_PORTD4 #define D5 eS_PORTD5 #define D6 eS_PORTD6 #define D7 eS_PORTD7 #define RS eS_PORTC6 #define EN eS_PORTC7
These connections must be defined for the proper working of the LCD library. Don’t forget to define these pins as Output.
Lcd8_Clear() & Lcd4_Clear() :Â Calling these functions will clear the 16×2 LCD display screen when interfaced with 8 bit and 4 bit mode respectively.
Lcd8_Set_Cursor() & Lcd4_Set_Cursor() : These function will set the cursor position on the LCD screen by specifying its row and column. By using these functions we can change the position of character and string displayed by the following functions.
Lcd8_Write_Char() & Lcd4_Write_Char() :Â These functions will write a single character to the LCD screen and the cursor position will be incremented by one.
Lcd8_Write_String() & Lcd8_Write_String() : These function will write string or text to the LCD screen and the cursor positon will be incremented by length of the string plus one.
Lcd8_Shift_Left() & Lcd4_Shift_Left() : This function will shift data in the LCD display without changing data in the display RAM.
Lcd8_Shift_Right() & Lcd8_Shift_Right() :Â This function will shift data in the LCD display without changing data in the display RAM.
8 Bit Mode Interfacing
Circuit Diagram
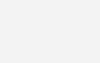
Atmel Studio C Code
#ifndef F_CPU #define F_CPU 16000000UL // 16 MHz clock speed #endif #define D0 eS_PORTD0 #define D1 eS_PORTD1 #define D2 eS_PORTD2 #define D3 eS_PORTD3 #define D4 eS_PORTD4 #define D5 eS_PORTD5 #define D6 eS_PORTD6 #define D7 eS_PORTD7 #define RS eS_PORTC6 #define EN eS_PORTC7 #include <avr/io.h> #include <util/delay.h> #include "lcd.h" //Can be download from the bottom of this article int main(void) { DDRD = 0xFF; DDRC = 0xFF; int i; Lcd8_Init(); while(1) { Lcd8_Set_Cursor(1,1); Lcd8_Write_String("electroSome LCD Hello World"); for(i=0;i<15;i++) { _delay_ms(500); Lcd8_Shift_Left(); } for(i=0;i<15;i++) { _delay_ms(500); Lcd8_Shift_Right(); } Lcd8_Clear(); Lcd8_Write_Char('e'); Lcd8_Write_Char('S'); _delay_ms(2000); } }
4 Bit Mode Interfacing
Circuit Diagram
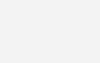
Atmel Studio C Code
#ifndef F_CPU #define F_CPU 16000000UL // 16 MHz clock speed #endif #define D4 eS_PORTD4 #define D5 eS_PORTD5 #define D6 eS_PORTD6 #define D7 eS_PORTD7 #define RS eS_PORTC6 #define EN eS_PORTC7 #include <avr/io.h> #include <util/delay.h> #include "lcd.h" //Can be download from the bottom of this article int main(void) { DDRD = 0xFF; DDRC = 0xFF; int i; Lcd4_Init(); while(1) { Lcd4_Set_Cursor(1,1); Lcd4_Write_String("electroSome LCD Hello World"); for(i=0;i<15;i++) { _delay_ms(500); Lcd4_Shift_Left(); } for(i=0;i<15;i++) { _delay_ms(500); Lcd4_Shift_Right(); } Lcd4_Clear(); Lcd4_Set_Cursor(2,1); Lcd4_Write_Char('e'); Lcd4_Write_Char('S'); _delay_ms(2000); } }
How to use the Header File?
You can download the lcd.h header file at the bottom of this article. Add the lcd.h to your project source group.
- Right Click on your project folder on the solution explorer on the right side.
- Add >> Existing Item
- Then Browse lcd.h
- Click Add
Optimize Code for More Efficiency
You already seen that by using our header file lcd.h, you can connect your 16×2 LCD to any of the output pins of the microcontroller. More coding is required for this feature which reduces the code efficiency and increases the size of hex file. You can solve this problem by making simple changes in the header file according to your LCD connections. For example consider above sample programs. I have used PORTD for sending data, 6th bit of PORTC as RS and 7th bit of PORTC as EN.
- Change Lcd4_Port(data) and Lcd8_Port(data) to PORTD = data
- Change pinChange(EN,1) to  PORTC |= (1<<PC7)
- Change pinChange(EN,0) to PORTC &= ~(1<<PC7)
- Change pinChange(RS,1) to PORTB |= (1<<PB6)
- Change pinChange(RS,0) to PORTC &= ~(1<<PC6)
You can download header file, atmel studio files and proteus files here…
Interfacing LCD with Atmega32 Microcontroller with Atmel Studio
You can try editing the header file as he mentioned. I think it shouldn’t take much time once you understand the code.
Can you please share it? I have the same question.
Hi Ligo, I modified de lcd.h code to work with AVR 162 internal oscillator 1MHz, It worked like a charm.
Thanks a lot
SIR !! HOW TO CONTROL WS2811 PIXEL LED USING ATMEGA328 IC !!!
Give the simple program for pattern design !!! please help me !!
Made a mistake in my previous comment:
It wasn’t supposed to be char *message[32], but char message[32].
In the first one, I’m declaring an array of pointers, but in the second one I’m declaring an array of characters which is what we want.
Try using the sprintf() function in the stdio.h library.
It converts the valu of any variable you give it into a string and then stores that string into char pointer.
Example:
char *message[32]; //32 because the display is 16×2
sprintf(message, “Value of variable: %d”, var);
Lcd8_Write_String(message); /*OR Lcd4_Write_String(message); if you’re using the LCD in 4-bit mode*/
Since you’re using an unsigned long, instead of writing %d you would write %lu.
Hope this helps!
I have already done it, it is only to understand a bit the program, the lcd.h file, it is known that the ATMEGA328p does not have port A by getting deletes everything that has to do with this file, modifies some “if” that are loose, and in the second part of the definition, place port B, this is the lcd.h, in the main you only have to change the pins in the definition and that’s it. luck
What are the changes that I must do to make it work in ATMEGA328P?, since it is the one that uses arduino I am interested in knowing how to handle it. Thank you very much for your attention.
Thanks, this is very useful.
One question, how can I print variables to the lcd? I have an unsigned long that I have no idea how to print on the screen. Thanks in advance
Awesome – thanks a lot (specially for wiring diagram and examples)
how can i run the program in atmega 328 with the header file.. what changes do i have to make in it ??
Thank you, very nice topic.
but i`m not found header file, where is header file?
i want 8bit header file….
thank you
can it work with atmega328p? I coudn`t do it…
Hi all
I have a few day working on the atmega2560 but i can work with the LCD, I don know which is the problem I follow the lcd.h(Peter Fleury) but it doesnt work I modified the F_CPU TO 16,8,4,1, i modified the MCU lline too in the makefile.
Someone had worked with it??
Thanks
Hey if i want to change
D7-C4 PIN1
D6-C6 PIN2
D5-C5 PIN3
D4-C4 PIN4
E-C2 PIN5
RS-C3 PIN6
WHAT should i do
Hi,
It is already descirbed above. Kindly check it.
what is difference between 4 bit and 8 bit mode of lcd ??
Just convert that value to string and display in LCD.
char temp,z,y;
if(a == 1)
{
temp = 0x80 + b;
z = temp>>4;
y = (0x80+b) & 0x0F;
Lcd4_Cmd(z);
Lcd4_Cmd(y);
}
else if(a == 2)
{
temp = 0xC0 + b;
z = temp>>4;
y = (0xC0+b) & 0x0F;
Lcd4_Cmd(z);
Lcd4_Cmd(y);
}
if(a == 3)
{
temp = 0x94 + b;
z = temp>>4;
y = temp & 0x0F;
Lcd4_Cmd(z);
Lcd4_Cmd(y);
}
else if(a == 4)
{
temp = 0xD4 + b;
z = temp>>4;
y = temp & 0x0F;
Lcd4_Cmd(z);
Lcd4_Cmd(y);
}
as you did?
Hello can i put changing string value??
some like :
i++;
Lcd4_Write_string(i);
thanks for help
Thank you very much for your precious help
You can take the header file and make necessary changes for it.
Hey ,
will this code work for atmega 2560 arduino also, if so who should i do it ?. I am using ardunio mega in which i dont have to use functions like liquidcrystal header file or any arduino functions. i am using embedded c.
Thanks for the feedback.
Hi,
Thanks for sharing this tutorial…It helped me a lot to up my 16×2 LCD display……I did some tweak but at last it was nice thanks for sharing……..
How did you do that?
Just convert that integer to string and display.
where they say: [You can download header file, atmel studio files and proteus files here…
Interfacing LCD with Atmega32 Microcontroller with Atmel Studio] the download link is highlighted
Where is download button ?????????????????????
is there any command
to show integer value on lcd?? plz me. i can’t show integer value
Check whether the program is running fine or not using simple led blinking.
i can use 4bit lcd interface with atmega16 and the below code on work in proteus but did not work in hardware. plz how to get the output in hardware.please suggested to me right know. all lcd pins connected to Uc properly
#include
#include
#include
#define rs PC1
#define rw PC2
#define en PC3
void lcd_init();
void dis_cmd(char);
void dis_data(char);
void lcdcmd(char);
void lcddata(char);
void LCD_str(unsigned char *p);
int main(void)
{
unsigned char data0[11]=”ENGINEERS”;
unsigned char data1[10]=”GARAGE”;
unsigned int adc_val;
int i=0;
DDRC=0xFF;
DDRA=0x00;
lcd_init();
ADC_init();
dis_cmd(0x01);
while(data0[i]!=”)
{
dis_data(data0[i]);
_delay_ms(50);
i++;
}
dis_cmd(0xC5);
i=0;
while(data1[i]!=”)
{
dis_data(data1[i]);
_delay_ms(50);
i++;
}
_delay_ms(1000);
while(1)
{
dis_cmd(0x83);
LCD_str(“ADC Value”);
dis_cmd(0xC3);
LCD_str(“temp:”);
adc_val=ADC_read(0x01);
adc_val=(adc_val*2500)/1024;
Set_LCD_Num(adc_val);
}
}
void lcd_init() // fuction for intialize
{
dis_cmd(0x02); // to initialize LCD in 4-bit mode.
dis_cmd(0x28); //to initialize LCD in 2 lines, 5X7 dots and 4bit mode.
dis_cmd(0x0C);
dis_cmd(0x06);
dis_cmd(0x83);
_delay_ms(1);
}
void dis_cmd(char cmd_value)
{
char cmd_value1;
cmd_value1 = cmd_value & 0xF0; //mask lower nibble because PA4-PA7 pins are used.
lcdcmd(cmd_value1); // send to LCD
_delay_us(50);
cmd_value1 = ((cmd_value<<4) & 0xF0); //shift 4-bit and mask
lcdcmd(cmd_value1); // send to LCD
_delay_us(50);
}
void dis_data(char data_value)
{
char data_value1;
data_value1=data_value&0xF0;
lcddata(data_value1);
_delay_us(750);
data_value1=((data_value<<4)&0xF0);
lcddata(data_value1);
_delay_us(750);
}
void lcdcmd(char cmdout)
{
PORTC=cmdout;//|0x08;
PORTC&=~(1<<rs);
PORTC&=~(1<<rw);
PORTC|=(1<<en);
_delay_ms(25);
PORTC&=~(1<<en);
}
void lcddata(char dataout)
{
PORTC=dataout;// | 0x0a;
PORTC|=(1<<rs);
PORTC&=~(1<<rw);
PORTC|=(1<<en);
_delay_ms(25);
PORTC&=~(1<<en);
}
//LCD STRING FUNCTION FOR PREFINED DATA
void LCD_str(unsigned char *p)
{
//unsigned char ch;
while((*p)!='')
{
//ch=*p;
dis_data(*p);
p++;
}
}
Take this as a reference : https://electrosome.com/custom-characters-lcd-pic-mplab-xc8/
how can i display the custom character on the lcd including time,date and temperature should be in degree celsius, but it is displaying all except degree celsius on lcd using avr microcontroller………..
Yes, you should add lcd.h header file wherever you are using LCD.
do we have to include lcd.h file every time we are using lcd , i.e. if we are going to do next project do we have to again use lcd.h as add file
I solved by my own:)
Hello can i put changing string value??
some like :
i++;
Lcd4_Write_string(i);
thanks for help
What you meant by variant ?
Yes, it should work.
hi. how i can put an variant in lcd8_write_string??????
Good morning, is it possibly connect displays pins
PD3 – D4
PD2 – D5
PD1 – D6
PD0 – D7
PD5 – RW
PD4 – E
Thank you for answers
while(1) is infinite loop…
Lcd8_Init() just initializes the LCD module in 8 bit interface mode.
hii…i’m the beginner and i have a question? what does it mean by lcd8-int(); functio? and what kind of loop it is while(1) ??
Hi
I want write another customized header file to interfacing with LCD
can you help me on this?
such as giving a source to learn more about that… 😉
Try reading the datasheet of HD44780 LCD Controller.
I have a 4×20 LCD how can i control the cursor as when i move it from line 1 to 2 by your function ,, i want to move it to line 3 and 4 ??
Hello, I haven’t the code for at32u3a0512….
but you can try modifying the above header file.. Logic is the same.. just change the output commands as it compatible with at32uc3a512..
hi…..
i need lcd libraray with at32uc3a0512 not atmega32….can you help me ?
Print it in a loop..
hey i want to scroll only a particular line, how to do…
I want to program for a Atmega32u4
But many errors :
Error 1 ‘PORTA’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 49 5 Leonardo32u4_LCD
Warning 2 each undeclared identifier is reported only once for each function it appears in c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 49 5 Leonardo32u4_LCD
Error 3 ‘PA0’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 49 19 Leonardo32u4_LCD
Error 4 ‘PA1’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 51 19 Leonardo32u4_LCD
Error 5 ‘PA2’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 53 19 Leonardo32u4_LCD
Error 6 ‘PA3’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 55 19 Leonardo32u4_LCD
Error 7 ‘PA4’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 57 19 Leonardo32u4_LCD
Error 8 ‘PA5’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 59 19 Leonardo32u4_LCD
Error 9 ‘PA6’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 61 19 Leonardo32u4_LCD
Error 10 ‘PA7’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 63 19 Leonardo32u4_LCD
Error 11 ‘PC0’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 81 19 Leonardo32u4_LCD
Error 12 ‘PC1’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 83 19 Leonardo32u4_LCD
Error 13 ‘PC2’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 85 19 Leonardo32u4_LCD
Error 14 ‘PC3’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 87 19 Leonardo32u4_LCD
Error 15 ‘PC4’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 89 19 Leonardo32u4_LCD
Error 16 ‘PC5’ undeclared (first use in this function) c:program filesatmelavr studio 5.1extensionsatmelavrgcc3.3.1.27avrtoolchainbin../lib/gcc/avr/4.5.1/../../../../avr/include/util/lcd.h 91 19 Leonardo32u4_LCD
WOW ?
as i used the code, i get delays as high as 5 secs between character shift
Hey George, I want to use 20×4 LCD module, what modification should I make?
Read the “Optimize Code for More Efficiency” section above and make changes to the header file accordingly..
hey , i want to create this program so that it only uses portd , not any other pins in port !! suggest the improvements , any change in header file of functions.
Which is the statement producing this error??
Warning 1 deprecated conversion from string constant to ‘char*’ [-Wwrite-strings]
I am using C++
HELP! 😉
Hello, your code is not using our library…
hello would you please help me troubleshoot this code i tried to interfaceATMEGA32A and programme it in 8bit lcd mode . it kept displaying the following message oohrhwhrhdhewhhww….., the code is as follows:
#include
#include
#define LCD_DPRT PORTB
#define LCD_DDDR DDRB
#define LCD_DPIN PINB
#define LCD_CPRT PORTA
#define LCD_CDDR DDRA
#define LCD_CPIN PINA
#define LCD_RS 0
#define LCD_RW 1
#define LCD_EN 2
void lcdCommand(unsigned char cmnd);
void lcd_data(unsigned char data);
void lcd_gotoxy(unsigned char x, unsigned char y);
void lcd_init(void);
void lcd_print(char *str);
void lcdCommand(unsigned char cmnd)
{
LCD_DPRT =cmnd;
LCD_CPRT &=~((1<<LCD_RS) |(LCD_RW));
_delay_us(1);
LCD_CPRT |=1<<LCD_EN;
_delay_us(1);
LCD_CPRT &=~(1<<LCD_EN);
_delay_us(10);
LCD_DPRT=0;
}
void lcd_data(unsigned char data)
{
LCD_DPRT =data;
LCD_CPRT |= 1<<LCD_RS;
LCD_CPRT &= ~ (1<<LCD_RW);
_delay_us(1);
LCD_CPRT |= (1<<LCD_EN);
_delay_us(1);
LCD_CPRT &= ~ (1<<LCD_EN);
_delay_us(10);
LCD_DPRT=0;
}
void lcd_init()
{
LCD_DDDR =0xFF;
LCD_CDDR =0xFF;
LCD_CPRT &=~(1<<LCD_EN);
_delay_us(2000);
lcdCommand(0x38);
// _delay_us(10);
lcdCommand(0x0E);
// _delay_us(10);
lcdCommand(0x01);
_delay_us(2000);
lcdCommand(0x06);
}
void lcd_gotoxy(unsigned char x, unsigned char y)
{
unsigned char firstCharAdr[]={0x80,0x0C};//,0x90,0xD4};
lcdCommand( 0x80 + firstCharAdr[y-1] + x-1);
_delay_us(100);
}
void lcd_print(char *str)
{
unsigned char i;
while(str[i] !=0)
{
lcd_data(str[i]);
i++;
}
}
int main(void)
{
lcd_init();
while(1)
{
lcd_gotoxy(1,1);
lcd_print("hello");
lcd_gotoxy(2,1);
lcd_print("world");
}
that’s a great library! thanks, man
how to download lcd.h file ?????????
plz send me the whole program of both 4 & 8 bit datalines………..
It is a mistake in the header file.. substitute the set cursor function for 4 bit with the following code..
void Lcd4_Set_Cursor(char a, char b)
{
char temp,z,y;
if(a == 1)
{
temp = 0x80 + b;
z = temp>>4;
y = (0x80+b) & 0x0F;
Lcd4_Cmd(z);
Lcd4_Cmd(y);
}
else if(a == 2)
{
temp = 0xC0 + b;
z = temp>>4;
y = (0xC0+b) & 0x0F;
Lcd4_Cmd(z);
Lcd4_Cmd(y);
}
}
Hi ! , how do I jump to row ??
Ok thanks for the feedback….please optimize the code before using it in hardware… otherwise it will consume more memory.. resulting in less efficiency..
I already find the problem, I was inverting the pins on define.
Here is the correct one:
#define DB4 eS_PORTB0
#define DB5 eS_PORTB1
#define DB6 eS_PORTB2
#define DB7 eS_PORTB3
#define RS eS_PORTB4
#define EN eS_PORTB5
Thx for all
HI I don’t understand the #define es_PORTXX Y on header, the Y waas supposed to be the pin number on datasheet? What the Y means?
I tried to change the programm to use as 4-bits LCD on ports:
D7-B0 PIN1
D6-B1 PIN2
D5-B2 PIN3
D4-B3 PIN4
E-B4 PIN5
RS-B5 PIN6
So I changed on program:
#define D4 eS_PORTD4
#define D5 eS_PORTD5
#define D6 eS_PORTD6
#define D7 eS_PORTD7
#define RS eS_PORTC6
#define EN eS_PORTC7
to
#define D4 eS_PORTB3
#define D5 eS_PORTB2
#define D6 eS_PORTB1
#define D7 eS_PORTB0
#define RS eS_PORTB4
#define EN eS_PORTB5
but it don’t work.
YOu should edit the CKSEL 3-0 bit.. for Calibrated internal RC oscillator as given in the datasheet..
What should I change to use the internal clock???
LCD.H header file is used to simplifying the code.. it makes the code more readable… and simple… I will explain the details of interfacing lcd with a microcontroller in one of posts..
The remaining 2 header files are the default header files of Atmel Studio..
whtat is the meaning of providing the code without all the header files.???
why those 2 header files are not included in download.????
where are the remaining 2 header files.????????????????????