Blinking LED using LPC2148 – ARM Microcontroller Tutorial – Part 3
Contents
Hello World
In this tutorial we will learn how to start programming an ARM microcontroller. This is a hello world project (blinking an LED) intended for beginners to ARM microcontroller programming. Here we are using LPC2148 ARM microcontroller and Keil IDE for programming.
Components Required
- LPC2148 Development Board
- LED
- 220R Resistor
Registers
In this section we will learn about different registers used for configuring or controlling a pin of an ARM microcontroller. In microcontrollers, pins are divided in to different PORTS. Usually a 32-bit microcontroller will have 32 pins per PORT (sometimes it may vary). We have 2 PORTS in LPC2148, P0 and P1. Each pin of these ports are named as P0.0, P0.1, P0.2, P1.0, P1.2 etc.
PINSELx
Usually most of the pins of an ARM microcontroller is multi-functional, every pin can be made to perform one of the assigned function by setting particular bits of PINSEL register.
Value | Function |
---|---|
00 | Primary Function (default), typically GPIO |
01 | First alternate function |
10 | Second alternate function |
11 | Third alternate function |
PINSEL registers for each pins.
Register | Pins |
---|---|
PINSEL0 | P0.0 to P0.15 |
PINSEL1 | P0.16 to P0.31 |
PINSEL2 | P1 |
Please refer the user manual of LPC2148 microcontroller for more details.
IOxDIR
This register is used to control the direction (input or output) of a pin, once is it configured as a GPIO pin (General Purpose Input Output) by using PINSELx register.
Value | Direction |
---|---|
0 | Input |
1 | Output |
IOxPIN
IOxPIN is GPIO port pin value register. This register is used to read the current state of port pins regardless of input or output configuration. And it is also used to write status (HIGH or LOW) of output pins.
IOxSET
IOxSET is GPIO output set register. This register is commonly used in conjunction with IOxCLR register described below. Writing ones to this register sets (output high) corresponding port pins, while writing zeros has no effect.
IOxCLR
IOxCLR is GPIO output clear register. As mentioned above, this register is used in conjunction with IOxSET. Writing ones to this register clears (output low) corresponding port pins, while writing zeros has no effect.
Keil C Code
#include "lpc214x.h" // Include LPC2148 header file #define LED_PIN 16 // Define LED to PIN 16 #define LED_PORT IO1PIN // Define LED to Port1 #define LED_DIR IO1DIR // Define Port1 direction register void delayMs(unsigned int x); int main() { PINSEL2 = 0x00000000; // Define port lines as GPIO LED_DIR |= (1 << LED_PIN); // Define LED pin as O/P LED_PORT &= ~(1 << LED_PIN); // Turn off the LED initially while(1) // Loop forever { LED_PORT |= (1 << LED_PIN); // Turn ON LED delayMs(1000); // 1 second delay LED_PORT &= ~(1 << LED_PIN); // Turn OFF LED delayMs(1000); // 1 second delay } return 0; } //Blocking delay function void delayMs(unsigned int x) { unsigned int j; for(;x>0;x--) for(j=0; j<0x1FFF; j++); }
Code Explanation
I hope that the above program is self explanatory as it is well commented, even though I am explaining few things below which may be confusing for beginners.
Expression | Equivalent | Explanation |
---|---|---|
<< | – | Left shift operator |
| | – | Bitwise OR operator |
& | – | Bitwise AND operator |
(1 << LED_PIN) | (1 << 16) | 00000000000000010000000000000000 |
LED_DIR |= (1 << LED_PIN) | IO1DIR = IO1DIR | (1 << 16) | Setting P1.16 as output pin |
LED_PORT &= ~(1 << LED_PIN) | IO1PIN = IO1PIN & ~(1 << 16) or IO1CLR = (1 << 16) | Setting P1.16 pin LOW |
LED_PORT |= (1 << LED_PIN) | IO1PIN = IO1PIN | (1 << 16) or IO1SET = (1 << 16) | Setting P1.16 pin HIGH |
Circuit Diagram
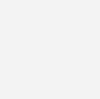
Circuit Description
LED is connected to pin 16 of port 1 via a current limiting resistor. And a 12MHz crystal is connected to the oscillator pins, which will provide the necessary clock for the operation of the microcontroller. We can use the UART lines as shown in the above circuit for flashing the chip using flash magic tool, please refer the article Flashing LPC2148 using Serial ISP Bootloader for more information about it.
If you are using a development board or an LPC2148 stick, you don’t need to worry much about circuit diagram. These will be already connected. Just make sure that the LED is connected to the pin provided in the C program.
Creating Keil Project
Hope you already downloaded and installed Keil IDE. You may refer this article for more details about installing Keil IDE.
- Open Keil IDE.
- Select Project >> New μVision Project.
- You can create a new folder and give a name of your choice for the new project as shown below.
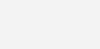
- Click Save.
- Next we need to select the microcontroller LPC2148.
- Click the drop down menu and select “Legacy Device Database” as shown below.
- Search for LPC2148 and select it.
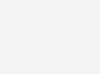
- Click OK.
- Next it should ask you for permission to add Startup.s file to your project as shown below.
- Click Yes.
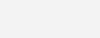
- Now you can see that the project is created.
- You can click + icon in the left side project folder section to see all the files in the project.
- Currently there is only one file, Startup.s .
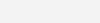
- Now we can add our source file to the project.
- Right click on “Source Group 1”.
- Select “Add New Item to Group ‘Source Group 1’ ” as shown below.
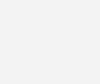
- Select C File.
- Give a name, for example “main.c”.
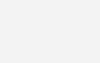
- Click Add.
- Now we can see that the new file is created.
- Now you can enter the code here and save it.
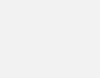
- Now we need to update few project settings.
- Click on the “Options for Target 1” icon as shown below.
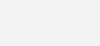
- Go to “Output” tab.
- Check the checkbox “Create Hex File” as shown below.
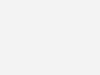
- Now go to “Linker” tab.
- Check the checkbox “Use Memory Layout from Target Dialog” as shown below.
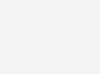
- Click OK.
- Now you can build the project using build button as shown below.
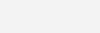
- Once the build is completed, make sure that there are no errors by checking the build output windows at the bottom.
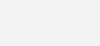
- Now the hex file should be generated in “Objects” folder inside your project folder as shown below.
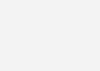
Flashing Program to the Chip
You can use a JTAG programmer (like ULINK 2, ULINK PRO, JLINK etc. ) or we can use on-chip ISP bootloader. Please read the article Flashing LPC2148 using On-Chip ISP Bootloader for more details.
Download Here
You can download the entire Keil project folder here.