Interfacing EEPROM with PIC Microcontroller
Contents
MikroC Programming
MikroC Pro for PIC Microcontroller provides built-in library routines to communicate with I2C devices. Here we deals only with Byte Write and Random Read operations. Using these you can easily make programs for other operations.
MikroC Function for Write Data to EEPROM :
void write_EEPROM(unsigned int address, unsigned int dat) { unsigned int temp; I2C1_Start(); // issue I2C start signal I2C1_Wr(0xA0); // send byte via I2C (device address + W) temp = address >> 8; //saving higher order address to temp I2C1_Wr(temp); //sending higher order address I2C1_Wr(address); //sending lower order address I2C1_Wr(dat); // send data (data to be written) I2C1_Stop(); // issue I2C stop signal Delay_ms(20); }
MikroC Function to Read Data from EEPROM :
unsigned int read_EEPROM(unsigned int address) { unsigned int temp; I2C1_Start(); // issue I2C start signal I2C1_Wr(0xA0); // send byte via I2C (device address + W) temp = address >> 8; //saving higher order address to temp I2C1_Wr(temp); //sending higher order address I2C1_Wr(address); //sending lower order address I2C1_Repeated_Start(); // issue I2C signal repeated start I2C1_Wr(0xA1); // send byte (device address + R) temp = I2C1_Rd(0u); // Read the data (NO acknowledge) I2C1_Stop(); return temp; }
Circuit Diagram for Demonstration :
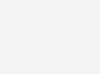
In this example we writes 00000001 to the first memory location, 00000010 to second, 000000100 to third etc sequentially up to 10000000. Then it is read sequentially and output through PORTB.
To simulate this project in Proteus you may need to connect I2C Debugger. SCL and SDA of I2C Debugger should be connected in parallel to SCL and SDA of 24C64. I2C Debugger can be found where CRO can be found in Proteus.
You can download the hex file, MikroC source code, Proteus files etc here…
Hi Ligo Sir
I am designing a speedometer cum odometer for my motor cycle.I need your help.My compiler is Mikro C.How can i store the odometer readings to external eeprom if the readings exceed 8 bit data?How can i store if the kilometer exceeds 255?Your earliest reply is expected.Give me the mikro c code also.Thanks in advance.I am using pic16f877a and 24c02 eeprom.Need to store km readings and read it back and display it on lcd(16×2).
hi,
please help me. how can i erase the contents of EEPROM 24LC512 in microc
if i will use 2 eeprom in the i2c bus.first address will be 1010000 and second device adress will be 1010001 ??
Sorry, I don’t have any C18 codes.
Hi Ligo, may I plz have the code for C18 compiler?? Actually i need to write a code for Library Management system by using PIC18F4550 microcontroller.
Yup..
oh, i got it now!u mean we hardwire the address of each EEPROMs by setting or grounding their A0,A1, A2 pins differently in 8 different combinations(since 3 bits are used), thus generating a particular address to each EEPROM, right? thanks a great deal
You can connect pins A0, A1, A2 of the eeprom to different status (VDD or VSS) … and you can address a particular eeprom as explained in the Device Addressing Section…
if we are connecting more than 1 EEPROMs then, (here since we can join 8 EEPROMs), how does the EEPROMs know that the a particular 3 bit code varying from 000 to 111 is meant for them?is there a code to assign those 3 bit codes to respective EEPROMs??
See the section Device Addressing…
You can see that there are 8bits for addressing…
Higher 4 bits (1010) is preset in the device.. next 3 bits are the status of A2, A1, A0 of the device and the last bit specifies whether we want to write or read from the device…….
By using A2,A1,A0 we can connect up to 8 EEPROM to an I2C Bus….
I2C1_Wr(0xA0); // send byte via I2C (device address + W)
what does this line do? specifically what is the ‘W’ here?