Getting Started with OpenWrt C Programing
Contents
In this tutorial we will learn how to cross compile a C program for OpenWrt. Cross compiling a C program for OpenWrt is a little complicated task for beginners. So I thought of writing a detailed tutorial for it. Please feel free to comment below if you have any doubts or you want to add anything more to this article.
Prerequisite
You need a linux system for making the OpenWrt build environment. We are using Ubuntu in this tutorial. Windows 10 users can enable and use Windows Subsystem for Linux, as I am doing here.
Installing Build Tools
Note : Do everything as non root user.
Here we are installing git and GNU build tools. We are installing svn (subversion) and mercurial as some of the feeds are not available over git.
sudo apt update sudo apt install git-core build-essential libssl-dev libncurses5-dev unzip gawk zlib1g-dev sudo apt install subversion mercurial
Collecting Environment Information
We need know the exact target environment before we make the build system and cross compiling. We can find the required information through following steps.
OpenWrt Version
Execute following command to get the version of OpenWrt.
cat /etc/banner
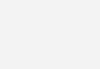
Please note my version is CHAOS CLAMER 15.05.1.
CPU Info
Get the CPU info by running following command.
cat /proc/cpuinfo
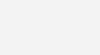
Please note my CPU is Atheros AR9330 rev 1 in TP-LINK TL-MR3020 board.
OpenWrt Build System
Downloading & Installing
First we need to download the OpenWrt source codes to make the build environment. I will be downloading chaos calmer. Please choose one depending on your target environment.
git clone https://github.com/openwrt/openwrt.git
OR
git clone https://github.com/openwrt/chaos_calmer.git
Go to the directory.
cd openwrt
OR
cd chaos_calmer
Then update and install feeds using following commands.
./scripts/feeds update -a ./scripts/feeds install -a
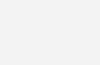
Configuring Target
Now you can configure the target environment by running following command.
make menuconfig
Then select Target System, Subtarget and Target Profile as per your target environment.
- Target System :Â Atheros AR7xxx/AR9xxx
- Subtarget : Generic
- Target Profile :Â TP-LINK TL-MR3020 (I am using TP-LINK MR3020 router)
Please make double sure that you are selecting these as per your target environment, otherwise the cross compiled binary file won’t be compatible with target environment.
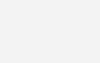
Building
Now we are ready for building the image. We can do it with a single command.
make
This may take several hours depending on the speed of your system. Please make sure that internet is available in your system during this process. By default make will run as many parallel jobs as possible, you may also specify it explicitly by using -j option.
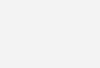
Once this is completed your system is ready for cross compiling.
Cross Compiling
Hope your machine is ready for cross compiling C program of OpenWrt. Before compiling the program we need to add all the necessary environment variables as explained below.
Make the Environment
For easy access we are storing all the environment variables in a bash script such that we can run the script whenever required. Create a file in bin folder using following command.
sudo nano /bin/openwrt.config
This will open nano text editor. You can copy below script and paste (right click) in your nano editor. Please don’t forget to change the toolchain directory location as per your download and install location.
# Set up paths and environment for cross compiling for openwrt export STAGING_DIR=/home/ligo/chaos_calmer/staging_dir export TOOLCHAIN_DIR=$STAGING_DIR/toolchain-mips_34kc_gcc-4.8-linaro_uClibc-0.9.33.2 export LDCFLAGS=$TOOLCHAIN_DIR/usr/lib export LD_LIBRARY_PATH=$TOOLCHAIN_DIR/usr/lib export PATH=$TOOLCHAIN_DIR/bin:$PATH
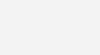
Now you can save and exit by pressing Ctrl+O and Ctrl+X respectively. Then setup the environment by running this script.
source /bin/openwrt.config
Once this is completed we can start programming.
Hello World Program
#include <stdio.h> int main() { printf("Hello World"); return 0; }
As you can see this is a simple program to print “Hello World”. Create a file called hello.c as shown below.
nano hello.c
Then copy the above program and paste (right click) in the editor.
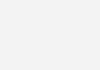
Now save and exit the editor by pressing Ctrl+O and Ctrl+X respectively.
Compile
Once the program is saved you can cross compile it using below command.
mips-openwrt-linux-gcc -o hello hello.c
Now you can see that the binary file hello is generated in the folder.
Testing
Next step is to test the generated binary file in the target environment. So connect your PC to your OpenWrt router. My router IP address is 192.168.4.1 . Here we are using scp (secure copy) for copying file from the PC to router.
scp hello [email protected]:hello
Enter the password. Once the file is copied, login to your router using ssh.
ssh [email protected]
Enter your password.
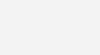
Once you logged in to your router, you can see that the binary file hello is there in the home folder. You can simply run it using following command.
./hello
You can see that “Hello World” message is printed in your terminal. If you are getting any other error message, it might be due to some mistake in above mentioned procedure. So please be careful in each and every step.
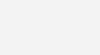
Hope you learned how to cross compile a C program for OpenWrt. Please feel free to comment below if you have any doubts.
Hi, I want to add a static library file when i compile. How would I do that?
Scroll up and see what is the exact error.
hi, when i do make -j 4 after some time i am getting make -r world: build failed
with Error 1.
i tried make -j1 V=s to print log