Led Blinking using Raspberry Pi – Python
Contents
Led blinking is one of the beginner circuits which helps one to get acquainted with GPIO pins of Raspberry Pi. Here we use Python language to write the code for blinking Led at one second intervals.
Components required
- One led
- 100 ohm resistor
- Jumper cables
Raspberry Pi GPIO Specifications
- Output Voltage : 3.3V
- Maximum Output Current : 16mA per pin with total current from all pins not exceeding 50mA
For controlling a Led using Raspberry Pi, both python and the GPIO library is needed.
Installing Python GPIO Library
Note:Â Python and GPIO library are preinstalled if you are using Raspbian.
- Make sure your Rasperry Pi is connected to the internet using either a LAN cable or a WiFi adapter.
- Open the terminal by double clicking the LXTerminal icon
- Type the following command to download the GPIO library as a tarball
wget http://raspberry-gpio-python.googlecode.com/files/RPi.GPIO-0.4.1a.tar.gz
- Unzip the tarball
tar zxvf RPi.GPIO-0.4.1a.tar.gz
- Change the directory to unzipped location
cd RPi.GPIO-0.4.1a
- Install the GPIO library in python
sudo python setup.py install
Raspberry Pi GPIO Pin Out
Raspberry Pi has 17 GPIO pins out of 26 pins
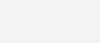
Circuit Diagram
- Connect the Led to 6 (ground) and 11 (gpio) with a 100Ω resistor in series
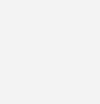
Python Programming
- Open Terminal
- Launch IDLE IDE by typing
sudo idle
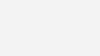
This launches IDLE with superuser privileges which is necessary execute scripts for controlling the GPIO pins
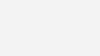
- After the IDLE launches, open a new window by FILE>OPEN or Ctrl+N
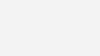
- Type the code below in the window
import time import RPi.GPIO as GPIO ## Import GPIO library GPIO.setmode(GPIO.BOARD) ## Use board pin numbering GPIO.setup(11, GPIO.OUT) ## Setup GPIO Pin 11 to OUT while True: GPIO.output(11,True) ## Turn on Led time.sleep(1) ## Wait for one second GPIO.output(11,False) ## Turn off Led time.sleep(1) ## Wait for one second
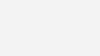
- Save the code by FILE>SAVE or Ctrl+S
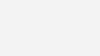
- To run your code RUN>RUN or Ctrl+F5
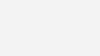
- The Led will start blinking now.
Good Idea,
You can try pinging to google or google dns (8.8.8.8 or 8.8.4.4) .
Try stackoverflow example.
http://stackoverflow.com/questions/20913411/test-if-an-internet-connection-is-present-in-python
Can we make it blink when no internet connection detected.? I want to use it for router restart when no internet.
Our small youtube channel : https://www.youtube.com/user/lijoppans/
Do you have youtube channel?