Using Push Button Switch with PIC Microcontroller
Contents
I hope that you have already read the first tutorial of Hi Tech C, LED Blinking using PIC Microcontroller. In that tutorial we blink LEDs connected to PORTB by writing to entire PORT and TRIS registers. In some cases we may want to set or reset individual bits of these registers. For that we can use the bit addressable feature of these registers. We have already seen in the previous tutorial that TRIS register is used to set the direction of each bits and PORT register is used to set the status of each bits in a port. A HIGH (1) at a bit of TRIS register makes the corresponding pin input and LOW (0) makes it output. In Hi-Tech C we can use following  methods to write to TRIS and PORT registers.
Prerequisites
Writing a Register
TRISB = 0x0F // In binary TRISB = 0b00001111 PORTB = 0xF0 // In binary PORTB = 0b11110000
Writing Bit
TRISB0 = 1 // Makes RB0 a input pin RB0 = 1 // Makes RB0 HIGH (VDD)
In this tutorial we will define a pin as input and another pin as output. A push button switch is connected to the input pin and the output pin drives an LED. When the switch is pressed, the LED glows for 2 seconds.
Circuit Diagram
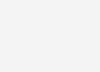
Pin RD0 is defined as an input pin, which is used to connect push button switch and pin RB0 is defined as an output pin, which drives an LED. RD0 is pull up to VDD using a 10KΩ resistor such that when the switch is not pressed the pin will be at the potential VDD and when the switch is pressed it will be grounded. A 470Ω resistor is connected in series with LED to limit the current through it.
Hi-Tech C Code
#include <htc.h> #define _XTAL_FREQ 8000000 void main() { TRISD0 = 1; //RD0 as Input PIN TRISB0 = 0; //RB0 as Output PIN RB0 = 0; //LED OFF do { if(RD0 == 0) //If Switch is presseed { __delay_ms(100); //Provides required delay if(RD0 == 0) //If Switch is still pressed { RB0 = 1; //LED ON __delay_ms(2000); //2 second delay RB0 = 0; //LED OFF } } }while(1); }
I hope that most parts of the above program are explained in its comments. 100ms delay between reading status of switch is provided for following reasons.
- The above program executes in 8MHz but the time period required for closing and opening the connection of switch varies from 100 – 300ms. Thus a delay must be provided while reading status of a switch. For eg: If you haven’t used delay while reading switch status which is used to counting, it will count multiple times with a single press.
- It will avoid false triggering by makes sure that the switch is being pressed after a delay.
- It will provide Switch Debouncing
Download Here
You can download Hi-Tech C files and Proteus files here…
yes, it is working but i am giving the connections RB0 is connected led to ground & RD0 is connect to the directly pull up to VDD & gnd
thank you sir
Greetings, sir. Before I ask, I would like to thank you for all the tutorials you’ve created and posted in this website.
I have a few question to ask regarding to my circuit in proteus. I’ve constructed the circuit you gave in the picture above. I also built the program using the hitech C compiler using Mplab software. And then, when I try to run the simulation in the proteus using the program i built, it didnt even say error or something like that, instead the led in the circuit itself wont light up. I tried to reinstall all my programming software (mplab, proteus, hitech c). it doesnt work. What should I do. This is really stressing me out. Your tutorials are the only source I have to learn about embedded programming.
Thank you.
Dear Vikram,
Kindly use our forums ( https://electrosome.com/forums/ ) for free technical support. Ping me on my email [email protected] if you need premium support.
hello sir i am using push button and LCD , so my task is how to display on off action of push button on ‘LCD when i press every time
means for 1 st press it should display on and second press it should display off , cycle repeats
plz tell me how to write c code for this my mail ID [email protected] Mob No 9028614218
Above example is 100% working ? Make sure that your oscillator frequency is 8MHz and Pull up resistor for the switch is there.
You can you MPLAB IDE for that. No need of any compilers like Hi-Tech C or XC8.
It is very simple. Declare a char variable and increment it during each key press. Write that character to PORTD.
PORTD = a;
hello sir george,i have two questions,i’m newbie in microntrollers, my first question is,if i have asm files what software will i use i will convert to HEX FILES?
NO.2,If i have HEX files coul i convert it to ASM files?
this quetion is really ppissing me off..some help pls admin?
by using 2 push buttons one for incrementing and other for decrementing firing angle of the triac (say in 9 steps one step 10 degrees)…can anybody please help me with code
THANK U
i want to control the firing angle of the triac in ac voltage controller by using a push button,please help me.
thank you
Yes. you are right.. I corrected it .
RB0 = 1 // makes 1st bit of portb high.., I think it is false
the correct is:
RB1=1, or makes 0st bit of portb high
Hello,
I don’t get your question. Please elaborate
do you mean: RB1=1 ?
why included pic.h in this code ???
Thanks for the suggestion… We will post it soon..
please post a eeprom tutorial (16f877a)
RB0 = 0 // makes 0th bit of portb low..
RB0 = 1 // makes 1st bit of portb high..
How to set input, output, high, law.. pin by pin in hi tech c?