Interfacing DC Motor with 8051 using L293D – AT89C51
Contents
In some of the electronics projects you may want to control a DC Motor with 8051 microcontroller. The maximum current that can be sourced or sunk from a 8051 microcontroller is 15 mA at 5v. But a DC Motor need currents very much more than that and it need voltages 6v, 12v, 24v etc, depending upon the type of motor used. Another problem is that the back emf produced by the motor may affect the proper functioning of the microcontroller. Due to these reasons we can’t connect a DC Motor directly to a microcontroller.
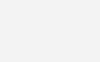
To overcome these problems you may use a H-Bridge using transistors. Freewheeling diodes or Clamp diodes should be used to avoid problems due to back emf. Thus it requires transistors, diodes and resistors, which may make our circuit bulky and difficult to assembly.
To overcome this problem the L293D driver IC is used. It is a Quadruple Half H-Bridge driver and it solves the problem completely. You needn’t connect any transistors, resistors or diodes. We can easily control the switching of L293D using a microcontroller. There are two IC’s in this category L293D and L293. L239D can provide a maximum current of 600mA from 4.5V to 36V while L293 can provide up to 1A under the same input conditions. All inputs of these ICs are TTL compatible and clamp diodes is provided with all outputs. They are used with inductive loads such as relays solenoids, motors etc.
L293D contains four Half H Bridge drivers and are enabled in pairs. EN1 is used to enable pair 1 (IN1-OUT1, IN2-OUT2) and EN2 is used to enable pair 2 (IN3-OUT3, IN4-OUT4). We can drive two DC Motors using one L293D, but here we are using only one. You can connect second DC Motor to driver pair 2 according to your needs.
Circuit Diagram
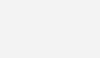
The DC Motor is connected to the first pair of drivers and it is enabled by connecting EN1 to logic HIGH (5V). VSS pin is used to provide logic voltage to L293D. Here 8051 microcontroller, which works at 5v is used to control L293D, hence the logic voltage is 5. The motor supply is given to Vs pin of the L293D.
Keil C Program
#include<reg52.h> #include<stdio.h> void delay(void); sbit motor_pin_1 = P2^0; sbit motor_pin_2 = P2^1; void main() { do { motor_pin_1 = 1; motor_pin_2 = 0; //Rotates Motor Anit Clockwise delay(); motor_pin_1 = 1; motor_pin_2 = 1; //Stops Motor delay(); motor_pin_1 = 0; motor_pin_2 = 1; //Rotates Motor Clockwise delay(); motor_pin_1 = 0; motor_pin_2 = 0; //Stops Motor delay(); }while(1); } void delay() { int i,j; for(i=0;i<1000;i++) { for(j=0;j<1000;j++) { } } }
Control Signals and Motor Status
P2.0/IN1 | P2.1/IN2 | Motor Status |
---|---|---|
LOW | LOW | Stops |
LOW | HIGH | Clockwise |
HIGH | LOW | Anti-Clockwise |
HIGH | HIGH | Stops |
You can download Keil C files and Proteus files here…
I want to run DC motor in Clockwise direction after 5ms Anti-clockwise direction after 10ms delay using timer1 mode1,AT89C51,L293D in C-Language to run in PROTES
I WANT TO RUN DC MOTOR IN CLOCKWISE & ANTI CLOCKWISE USING AT89C51 AND L293D WITHOUT USING ANY SWITCH , BY THE HELP OF DELAY CAN I RUN IT IN PROTEUS IN C LANGUAGE.
IF IT PLEASE SEND ME THE PROGRAM..
If you want to rotate to specific angles, go for servo motor or stepper motor.
Include TSOP1738 and sense TV remote signals.
You can use 2 L293D for that..
what will be the default rotation angle of the DC motor while rotating CW and CCW ??
if I want to rotate it to 45 degrees, what should I do??????????
try using LM358 window comparator.
hey, i want to control 3 dc motors using a micro controller,so what should i do?
i
i wanted to do the same so i edited the above given code and it works just fine ….. here’s the code
#include
#include
void delay(void);
void Aclock(void);
void clock(void);
void stopone(void);
void stopzero(void);
sbit motor_pin_1 = P2^0;
sbit motor_pin_2 = P2^1;
sbit sw_1 = P1^0;
sbit sw_2 = P1^1;
void main()
{
do
{
if(sw_1 == 0 && sw_2 == 1)
{ Aclock();}
else if(sw_1 == 1 && sw_2 == 0)
{clock();}
else if(sw_1 == 1&& sw_2 == 1)
{
stopone();
}
else{
stopzero();
}
}while(1);
}
void Aclock()
{
motor_pin_1 = 1;
motor_pin_2 = 0; //Rotates Motor Anit Clockwise
}
void clock()
{
motor_pin_1 = 0;
motor_pin_2 = 1; //Rotates Motor Clockwise
}
void stopone()
{
motor_pin_1 = 1;
motor_pin_2 = 1; //Stops Motor
}
void stopzero(){
motor_pin_1 = 0;
motor_pin_2 = 0; //Stops Motor
}
void delay()
{
int i,j;
for(i=0;i<1000;i++)
{
for(j=0;j<1000;j++)
{
}
}
}
how can i rotate the 2 dc motor tv remote in this circuit ?
You can use a bluetooth module like HC-05 or HC-06 for that purpose.
For controlling the speed of dc motor you may need to use PWM modules.
I want to control 8 motor with different speed and bidirection also 8051 Development Board
Just connect and program it to different pins of the microcontroller.
i want tom drive theree moters in different time what shall i do
Yes, you can replace it.
You can even use power MOSFETs for high power. You may add opto isolators also.
Which driver should i use for high power motor of order of 100w??
can i replace L293D by RKI 1340??
ill help you with that
Sorry, I don’t have.
can u give the assembly language program for this
You can use comparator for that.
Delay will depend on the speed of the motor you are using.
i want to rotate dc motor in clockwise for 1 full cycle. how much delay should i give for it i m using arm controller with L293d motor driver..can u give me program code for delay only for one and also for half cylce rotation
Sorry we don’t have any assembly codes.
i need dc motor direction interfacing code in assembly language
by using 5 switches
1 switch for clock wise if switch on , if off then motor become off
1 switch for anti-clock wise if switch on , if off then motor become off
1 switch for anti-clock wise continue rotation if switch on
1 switch for anti-clock wise continue rotation if switch on
1 switch for stop the rotation
Hello,
You can use the any USB to UART converter (supported by your OS) for that..
Use the component COMPIM in proteus.
It is very simple… clockwise and anticlockwise are already explained above…
Please read the following article to know how to read the status of a switch.
https://electrosome.com/8051-keilc-push-button-switch-at89c51/
Is it because I am just using same 5v in 8th no. pin of L293D??
I used at89s52 assembled the whole circuit in bread board but didn’t worked out. I double-triple checked the connection but didn’t worked out.
FYI-Proteus file is working perfectly.
Helloo..,
I’ve the question that can I use Atmega8 processor in place of mentioned in the ckt diagram.?
And the programm will be same for it..?
You can use any AT89C51 programmer..
I think you used a serial programmer… which is connected to PC via a USB to UART Converter…
So I can use any programmer? It is not necessary to convert the logic level to rs232?
All the programmers use the spi pins ,so is it that the programming doesnt change in anyway?
You can write program to microcontroller by using a programmer…
How are you supposed to load the program onto the microcontroller(as it is without any developer board)?
In proteus or hardware??
Try stopping the motor 1 or 2 seconds before rotating it in other direction as motor has inertia…
i want to operate a dc motor using 2 switches. i.e; if i press sw1 it moves in clockwise direction ,and if i press sw2 it moves in anticlockwise direction. but they are still running in both the cases 🙁 they won’t stop at all .what to do ?