Interfacing EEPROM with PIC Microcontroller
Contents
MikroC Programming
MikroC Pro for PIC Microcontroller provides built-in library routines to communicate with I2C devices. Here we deals only with Byte Write and Random Read operations. Using these you can easily make programs for other operations.
MikroC Function for Write Data to EEPROM :
void write_EEPROM(unsigned int address, unsigned int dat) { unsigned int temp; I2C1_Start(); // issue I2C start signal I2C1_Wr(0xA0); // send byte via I2C (device address + W) temp = address >> 8; //saving higher order address to temp I2C1_Wr(temp); //sending higher order address I2C1_Wr(address); //sending lower order address I2C1_Wr(dat); // send data (data to be written) I2C1_Stop(); // issue I2C stop signal Delay_ms(20); }
MikroC Function to Read Data from EEPROM :
unsigned int read_EEPROM(unsigned int address) { unsigned int temp; I2C1_Start(); // issue I2C start signal I2C1_Wr(0xA0); // send byte via I2C (device address + W) temp = address >> 8; //saving higher order address to temp I2C1_Wr(temp); //sending higher order address I2C1_Wr(address); //sending lower order address I2C1_Repeated_Start(); // issue I2C signal repeated start I2C1_Wr(0xA1); // send byte (device address + R) temp = I2C1_Rd(0u); // Read the data (NO acknowledge) I2C1_Stop(); return temp; }
Circuit Diagram for Demonstration :
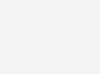
In this example we writes 00000001 to the first memory location, 00000010 to second, 000000100 to third etc sequentially up to 10000000. Then it is read sequentially and output through PORTB.
To simulate this project in Proteus you may need to connect I2C Debugger. SCL and SDA of I2C Debugger should be connected in parallel to SCL and SDA of 24C64. I2C Debugger can be found where CRO can be found in Proteus.
You can download the hex file, MikroC source code, Proteus files etc here…