Digital Thermometer using PIC Microcontroller and LM35 Temperature Sensor
Contents
Basics
A Digital Thermometer can be easily constructed using a PIC Microcontroller and LM35 Temperature Sensor. LM35 series is a low cost and precision Integrated Circuit Temperature Sensor whose output voltage is proportional to Centigrade temperature scale. Thus LM35 has an advantage over other temperature sensors calibrated in Kelvin as the users don’t require subtraction of large constant voltage to obtain the required Centigrade temperature. It doesn’t requires any external calibration. It is produced by National Semiconductor and can operate over a -55 °C to 150 °C temperature range. Its output is linearly proportional to Centigrade Temperature Scale and it output changes by 10 mV per °C.
The LM35 Temperature Sensor has Zero offset voltage, which means that the Output = 0V, at 0 °C. Thus for the maximum temperature value (150 °C), the maximum output voltage of the sensor would be 150 * 10 mV = 1.5V. If we use the supply voltage (5V) as the Vref+ for Analog to Digital Conversion (ADC) the resolution will be poor as the input voltage will goes only up to 1.5V and the power supply voltage variations may affects ADC output. So it is better to use a stable low voltage above 1.5 as Vref+. We should supply Negative voltage instead of GND to LM35 for measuring negative Temperatures.
This article only covers the basic working of Digital Thermometer using PIC Microcontroller and LM35, and uses 5V as Vref+. If you want more accurate results it is better to select Vref+ above 2.2V. I suggest you to use MCP1525 IC manufactured by Microchip, which will provide precise output voltage 2.5.
Suggested Readings:
Circuit Diagram
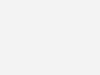
Note: VDD and VSS of the pic microcontroller is not shown in the circuit diagram. VDD should be connected to +5V and VSS to GND.
You can download the complete MikroC Source Code and Proteus files at the bottom of this post. The Analog output voltage of LM35 temperature sensor is given to the Analog Input pin AN0 of the PIC Microcontroller. The result of the 10-bit Analog to Digital (A/D) Conversion is read using the function ADC_Read(0). This 10-bit digital value is then converted to the corresponding voltage by multiplying with 0.4887 (For More Details read: Analog to Digital Converter in PIC Microcontroller). Then the Voltage is converted to corresponding character to Display it in LCD.
MikroC Code
// LCD module connections sbit LCD_RS at RB2_bit; sbit LCD_EN at RB3_bit; sbit LCD_D4 at RB4_bit; sbit LCD_D5 at RB5_bit; sbit LCD_D6 at RB6_bit; sbit LCD_D7 at RB7_bit; sbit LCD_RS_Direction at TRISB2_bit; sbit LCD_EN_Direction at TRISB3_bit; sbit LCD_D4_Direction at TRISB4_bit; sbit LCD_D5_Direction at TRISB5_bit; sbit LCD_D6_Direction at TRISB6_bit; sbit LCD_D7_Direction at TRISB7_bit; // End LCD module connections int t; char a; char lcd[] = "000 Degree"; void main() { ADCON1 = 0x04; Lcd_Init(); Lcd_Cmd(_LCD_CURSOR_OFF); do { Lcd_Cmd(_LCD_CLEAR); Lcd_out(1,1, "Temperature:"); t = ADC_Read(0); t = t * 0.4887; a = t%10; lcd[2] = a + '0'; t = t/10; a = t%10; lcd[1] = a + '0'; t = t/10; a = t%10; lcd[0] = a + '0'; Lcd_out(2,1,lcd); Delay_ms(100); }while(1); }
Download Here
You can download the MikroC Source Code and Proteus files etc from here.
If your temperature won’t cross 3 digits, use 2 digits only… You may even add decimal point if you like.
t = t/10;
a = t%10;
lcd[0] = a + ‘0’;
Is it okay if I don’t add this extra third lcd[0]?
You should make required changes to above program.
Hello sir how can I use this given code to construct an overheat detector rather than a thermometer. Except that the led display is interchanged with a buzzer and an LED.
hello.. what the app i need to open the file cording?
sir,
a = t%10;
lcd[2] = a + ‘0’;
what does this mean?
is that [2] means the units place?
can you tell me, how to display negative(minus) temperature in LCD using LM35 and PIC 16f….?
To convert variable a to a character.
lcd[0] = a + ‘0’;
what is the purpose of adding character ‘0’?
Please use our forums ( https://electrosome.com/forums ) for asking doubts outside the scope of above article.
Please see the ADCON1 register in the datasheet.
You can use simple if() in standard c for comparisons.
how will be the code written if you add a MQ-2 smoke sensor to the circuit???….
In the above program u have assigned RA0 as analog input and rest of them are digital inputs.. i want to assign RA3 as analog input instead of RA0. can u please help me out this..
how can i make comparison between two values of temperature with a reference value,, so that if it exceed the ref value, then should display HIGH TEMP and if it is below the ref value should display LOW TEMP
You can use a GPRS, Ethernet or WiFi modules for that.
how to send temperature data to internet
I hope that you can use it with a serial resistor connected to the supply. Just read voltage across the NTC and then you can easily convert it to temperature.
Hello sir
you are using LM35 sansor. but i want to use thermistor (NTC) 10K. Can you tell me how to calculate for Temperature.
what should i do if i want to use crystal 20mhz
Thank you sir for this post. It really helps me a lot on my project.
I’ll comment here too, If I have some problems encountered.
why we use L293D ic to use motor?
Yes, you can. Just change the PIC in MIkroC Project Settings.
It is better to change all PORT registers to LAT registers in OUTPUT OPERATIONS.
Hi, can i exchange the pic16f877A to pic 18f4520?
You can download the complete project files above.
what is the code?
how can i do this project with a keyboard 4×3 ?
https://electrosome.com/multiplexing-seven-segment-displays/
hi! can you please help me to create using 7segment display instead of LCD?? please??
ADCON1 defines some pins as digital and some as analog.. Please read the datasheet….
No need to change ADCON1 ..
sir could u tell me why you made adcon1 to 0x04..and should i make any change if i use a 16mhz crystal..???
Try using ADCON1 = 0x02;
HI I HAVE PROBLEM I WANT TO AFFICHER TOW VALEUR OF TEMPERATURE BUT PROGRAMME NOT WORK
// LCD module connections
sbit LCD_RS at RB4_bit;
sbit LCD_EN at RB5_bit;
sbit LCD_D4 at RB0_bit;
sbit LCD_D5 at RB1_bit;
sbit LCD_D6 at RB2_bit;
sbit LCD_D7 at RB3_bit;
sbit LCD_RS_Direction at TRISB4_bit;
sbit LCD_EN_Direction at TRISB5_bit;
sbit LCD_D4_Direction at TRISB0_bit;
sbit LCD_D5_Direction at TRISB1_bit;
sbit LCD_D6_Direction at TRISB2_bit;
sbit LCD_D7_Direction at TRISB3_bit;
float volt,temp;
unsigned char volt_char[15];
float volt1,temp1;
unsigned char volt1_char[15];
void main()
{
ADCON1 = 0b11;
PORTA = 0;
TRISA = 0X0F;
PORTB = 0;
TRISB = 0;
PORTC = 0;
TRISC = 0;
PORTD = 0;
TRISD = 0XFF;
lcd_init() ;
lcd_cmd(_lcd_clear);
lcd_cmd(_lcd_cursor_off);
lcd_out(1,3,”temp = “);
lcd_out( 1,18,”°c”);
lcd_out(1,3,”temp1 = “);
lcd_out( 1,18,”°c”);
ADC_Init();
while(1)
{ volt=adc_read(0);
volt1=adc_read(1);
volt=volt/1024;
volt=volt*500;
temp=volt/10;
floattostr(temp,volt_char);
lcd_out(1,10,volt_char);
delay_ms(1000);
lcd_cmd(_lcd_clear);
lcd_out(1,3,”temp = “);
lcd_out(1,18,”°c”);
delay_ms(1000);
volt1=volt1/1024;
volt1=volt1*500;
temp1=volt1/10;
floattostr(temp1,volt1_char);
lcd_out(2,10,volt1_char);
delay_ms(1000);
lcd_cmd(_lcd_clear);
lcd_out(2,3,”temp1 = “);
lcd_out(2,18,”°c”);
}
}
Hello,
This commenting section is to ask doubts about above article.. You can use our forums ( https://electrosome.com/forums ) for free support outside the scope of above article…
And we are not able to provide instant reply for free support….
You can program it depending upon your logic… use if case.. etc.
Voltage Sensor ?
Above article is about Thermometer..
Please use our forums : https://electrosome.com/forums for asking doubts not realted to above article.
You can use the Trobleshoot option in the pickit2 too..
Please comment dobuts in the corresponding article.
plzzzzzzzzzzzzzzzzz help
i want to work deux pomp but progra,,e not work
sbit LCD_RS at RB4_bit;
sbit LCD_EN at RB5_bit;
sbit LCD_D4 at RB0_bit;
sbit LCD_D5 at RB1_bit;
sbit LCD_D6 at RB2_bit;
sbit LCD_D7 at RB3_bit;
sbit LCD_RS_Direction at TRISB4_bit;
sbit LCD_EN_Direction at TRISB5_bit;
sbit LCD_D4_Direction at TRISB0_bit;
sbit LCD_D5_Direction at TRISB1_bit;
sbit LCD_D6_Direction at TRISB2_bit;
sbit LCD_D7_Direction at TRISB3_bit;
unsigned long temp;
unsigned int vDisp[3];
unsigned char Display[7];
float tempcomp;
void main() {
tempcomp = 30 ;
PORTC = 0;
TRISC = 0;
PORTD = 0;
TRISD = 0X08;
PORTA = 0;
TRISA = 0X01;
PORTB = 0;
TRISB = 0;
LCD_Init();
LCD_Cmd(_LCD_CURSOR_OFF);
LCD_Cmd(_LCD_CLEAR);
LCD_Out(1, 1, “Temp = “);
//Display = “+125 ‘C”;
Display[4] = 39; //’
Display[5]= ‘C’;
ADCON1 = 0x0E;
ADC_Init();
while (1){
temp = (ADC_Get_Sample(0) * 500) >> 10;
vDisp[0] = temp / 100;
vDisp[1] = (temp / 10) % 10;
vDisp[2] = temp % 10;
Display[1] = vDisp[0] + 48;
Display[2] = vDisp[1] + 48;
Display[3] = vDisp[2] + 48;
LCD_Chr(1, 8, Display[0]);
LCD_Chr(1, 9, Display[1]);
LCD_Chr(1, 10, Display[2]);
LCD_Chr(1, 11, Display[3]);
LCD_Chr(1, 12, Display[4]);
LCD_Chr(1, 13, Display[5]);
//LCD_Out(1, 8, ); // ‘Show temperature
if((temp > tempcomp)&& ((portd.f0==1) && (portd.f1==1))&&((portd.f2==0) && (portd.f3==0)))
{
portc=0X01;
}
if((temp < tempcomp)&& ((portd.f0==0) && (portd.f1==0))&&((portd.f2==1) && (portd.f3==1)))
{
portc=0X02;
}
if((temp == tempcomp)&& ((portd.f0==1) && (portd.f1==1))&&((portd.f2==1) && (portd.f3==0)))
{ portc=0X00;
}
delay_ms(200); //200ms delay for waiting
}
}
What will the project look like if add a bit sound for abnormal conditions?
How will the project look like if I add a bit sound for abnormal conditions?
What would the project looks like if I add a bit sound for abnormal conditions?
may i ask you about voltage sensor? what type of voltage sensor can i use for data logger? please help me
How can we understand PICKIt2 is working properly or not?
You should make those pins output by writing to TRIS register.
Hey man, nice job! I have a problem. When I try to connect a led bar in the D gate, when more than one pin is activated they turn gray insted of red and only the last one in the code is activated. Any Ideas ?
tnkz
8MHz
crystal value plz
Actually I don’t get your question….
To display adc result in LCD, you should convert it to string….. you may use mikroc’s IntToStr() or make a custom function..
sir , after getting ADC result on particuler integer like “temp”…then what is the next procedure to convert it in hundred, tens then unit.then display it on lcd …i am using mikroc compiler…pleas help i wana understand how ADC work
It will not blink so much in hardware….. You may remove lcd clear command from the loop.. or you can increase the loop delay.
sir, in software simulation LCD is blinking , is it possible for hardware to blink? and what should i do if this happen?
Sorry, I don’t have assembly code.
Can you send me an email containing the full schematic diagram of the whole circuit and a copy of code in assembly. Having a hard time to make an assembly code. I’m using C language but my professor required to use assembly language. Please help me I’m in the verge of my grade. [email protected] please eail me as soon as possible.
You should connect output of LM35 to a pin of PIC which is configured as Analog Input.
I found loading effect while connecting LM35 output directly to PIC Pa0 of 16F676.
CAN ANYONE CAN give clue to solution
I don’t get what are your doubts. You can make this circuit on hardware. It will work without any problems.
hi Mr. Lego
sir i want to build this project in hardware, and this is not my first time making electronics(but still very beginner ), can you give me instructions? like where to connect the real battery(5volts) and etc,, i had hard time interpreting the schematic to actual connection,, and i notice when i run this project in proteus when LM35 reads 80 the out on LCD display was 8 celcuis.(can you explain a bit sir.)… i really need your advise on this…
and by the way tnx for the open source project,,
my email if in case–>> [email protected]
what code can i use to make rc2/ccp1 as output….to make that rc2 ccp1 have voltage…………………………..at maplab use this
PR2 = 155; //Set the PWM period at PR2 (PR2=0 until 255)
CCPR1L = 0b10001100; //Set the duty cycle (CCPR1L = 8MSb)
CCP1CONbits.CCP1X = 1; //Set the duty cycle (CCP1Y=Bit5,LSb)
CCP1CONbits.CCP1Y = 0; //Set the duty cycle (CCP1X=Bit4,LSb)
TRISCbits.TRISC2 = 1; //Setting RC2 as output
T2CONbits.T2CKPS = 0b10; //Setting TMR2 Prescale as 16
T2CONbits.TMR2ON = 1; //Enable the CCP1 (RC2) PWM Mode
CCP1CONbits.CCP1M = 0b1100; //Setting RC2(CCP1) as PWM Mode
Yes, you can add dc motor.. don’t connect motor directly to microcontroller.. use drivers… eg : L293D.
Sorry I haven’t mplab x code..
y i cant post picture..zzz
zz
hi cant help me, i want to add mini pump water, and i switch pump to dc motor 12v, wt code can i add to make my dc motor working at specific temperature…can u give me file c for mplab x ide…
Try multiplexing of seven segment displays.. Try the following links :
http://electrosome.com/multiplexing-seven-segment-displays/
Sir I am New to this …
How can I re configure this in to Seven Segment display… ( 3 Digit with 1 Decimal Place )
Thank You
Of-course there will be some error.. as the result will be truncated to nearest integer……. But it will provide enough accuracy that we needed..
Ligo George,Please explain you declared “t” as ïnt” , but you multipled it with 0.4887 which is a real number, how can it gives an error less result
I haven’t yet used it. .
You can use com port functions //
The above circuit will work correctly…
Don’t forget to connect VDD and VSS of PIC
i forgot this image
hello, i have made few modifications on the isis file and the simulation in the isis run successfuly but the circuit doesn’t work
SIR DO YOU CODES IN INTERFACING THE MICROCONTROLLER TO VISUAL STUDIO USING UART??
Sir, i want to ask is it work well ? And you use LM35CZ or LM35DZ ?
t = t * 0.4887;
this is to convert the adc value to corresponding voltage…. see our ADC tutorial..
all other steps are to convert Voltage to corresponding string.
Bro, can you please explain these lines:
t = t * 0.4887;
a = t%10;
lcd[2] = a + ‘0’;
t = t/10;
a = t%10;
lcd[1] = a + ‘0’;
t = t/10;
a = t%10;
lcd[0] = a + ‘0’;
It is a good practice to avoid floating point calculations… as the microcontroller haven’t a floating point unit……..Floating point calculation require more clock cycle to complete its operation.
can we declare ‘t’ as float direcly? instead of declaring ‘t’ as ‘int’ and ‘a’ as ‘char’ and doing calculations?
Yes you can do it… Feed the amplified mic signal to an analog input of pic.. when the analog voltage increases above a limit you can turn lcd on..
Hi. I am trying to integrate this circuit with a clap switch circuit. So when i clap, lcd turn on and clap again, lcd off. However, clap switch is pic12. Can it work?
It is 8MHz… if you want the change the frequency.. just change it in mikroc project setting and compile..
what is the value of the crystal used in this project?
If i am using 8MHz, any changes to the program?
Lcd_Out(2,1, “hai”);
prints hai on the 2nd line..
Sir, how to make the LCD to start at second row?
Check their datasheets… There are some differences..
what are the differences sir?
There are some differences…
Sir, are pic16f877 and pic16f877a totally different?
It is used to adjust the contrast of the LCD.. . It is better to use a preset…
Dear Sir, what is the purpose of variable resistor RV1?
Is it ok if I connect VEE of LCD display to ground?
Sorry, I don’t know..
sir, besides Proteus, is there any equivalent software that can be used to open the Proteus files?
This is not an MPLAB project.. Use MikroC Pro..
sir, when i try to build the project in mplab ide, it failed to build:
Error[113] DIGITAL THERMOMETERDT.ASM 7 : Symbol not previously defined (ADCON1)
Error[152] DIGITAL THERMOMETERDT.ASM 7 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 12 : Symbol not previously defined (FARG_Lcd_Cmd_out_char)
Error[152] DIGITAL THERMOMETERDT.ASM 12 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 13 : Symbol not previously defined (_Lcd_Cmd)
Error[152] DIGITAL THERMOMETERDT.ASM 13 : Executable code and data must be defined in an appropriate section
sir, when i try to build the project in mplab ide, it failed to build:
Error[150] DIGITAL THERMOMETERDT.ASM 2 : Labels must be defined in a code or data section when making an object file
Error[152] DIGITAL THERMOMETERDT.ASM 6 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 7 : Symbol not previously defined (ADCON1)
Error[152] DIGITAL THERMOMETERDT.ASM 7 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 9 : Symbol not previously defined (_Lcd_Init)
Error[152] DIGITAL THERMOMETERDT.ASM 9 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 11 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 12 : Symbol not previously defined (FARG_Lcd_Cmd_out_char)
Error[152] DIGITAL THERMOMETERDT.ASM 12 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 13 : Symbol not previously defined (_Lcd_Cmd)
Error[152] DIGITAL THERMOMETERDT.ASM 13 : Executable code and data must be defined in an appropriate section
Error[150] DIGITAL THERMOMETERDT.ASM 15 : Labels must be defined in a code or data section when making an object file
Error[152] DIGITAL THERMOMETERDT.ASM 17 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 18 : Symbol not previously defined (FARG_Lcd_Cmd_out_char)
Error[152] DIGITAL THERMOMETERDT.ASM 18 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 19 : Symbol not previously defined (_Lcd_Cmd)
Error[152] DIGITAL THERMOMETERDT.ASM 19 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 21 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 22 : Symbol not previously defined (FARG_Lcd_Out_row)
Error[152] DIGITAL THERMOMETERDT.ASM 22 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 23 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 24 : Symbol not previously defined (FARG_Lcd_Out_column)
Error[152] DIGITAL THERMOMETERDT.ASM 24 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 25 : Symbol not previously defined (?lstr1_dt)
Error[152] DIGITAL THERMOMETERDT.ASM 25 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 26 : Symbol not previously defined (FARG_Lcd_Out_text)
Error[152] DIGITAL THERMOMETERDT.ASM 26 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 27 : Symbol not previously defined (_Lcd_Out)
Error[152] DIGITAL THERMOMETERDT.ASM 27 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 29 : Symbol not previously defined (FARG_ADC_Read_channel)
Error[152] DIGITAL THERMOMETERDT.ASM 29 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 30 : Symbol not previously defined (_ADC_Read)
Error[152] DIGITAL THERMOMETERDT.ASM 30 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 31 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 31 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 32 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 32 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 33 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 33 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 34 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 34 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 36 : Symbol not previously defined (_Int2Double)
Error[152] DIGITAL THERMOMETERDT.ASM 36 : Executable code and data must be defined in an appropriate section
Warning[202] DIGITAL THERMOMETERDT.ASM 37 : Argument out of range. Least significant bits used.
Error[152] DIGITAL THERMOMETERDT.ASM 37 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 38 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 38 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 39 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 40 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 40 : Executable code and data must be defined in an appropriate section
Warning[202] DIGITAL THERMOMETERDT.ASM 41 : Argument out of range. Least significant bits used.
Error[152] DIGITAL THERMOMETERDT.ASM 41 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 42 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 42 : Executable code and data must be defined in an appropriate section
Warning[202] DIGITAL THERMOMETERDT.ASM 43 : Argument out of range. Least significant bits used.
Error[152] DIGITAL THERMOMETERDT.ASM 43 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 44 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 44 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 45 : Symbol not previously defined (_Mul_32x32_FP)
Error[152] DIGITAL THERMOMETERDT.ASM 45 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 46 : Symbol not previously defined (_Double2Int)
Error[152] DIGITAL THERMOMETERDT.ASM 46 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 47 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 47 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 48 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 48 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 49 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 49 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 50 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 50 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 52 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 53 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 53 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 54 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 55 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 55 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 56 : Symbol not previously defined (_Div_16x16_S)
Error[152] DIGITAL THERMOMETERDT.ASM 56 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 57 : Symbol not previously defined (R8)
Error[152] DIGITAL THERMOMETERDT.ASM 57 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 58 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 58 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 59 : Symbol not previously defined (R8)
Error[152] DIGITAL THERMOMETERDT.ASM 59 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 60 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 60 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 61 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 61 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 62 : Symbol not previously defined (_a)
Error[152] DIGITAL THERMOMETERDT.ASM 62 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 64 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 65 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 65 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 66 : Symbol not previously defined (_lcd)
Error[152] DIGITAL THERMOMETERDT.ASM 66 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 68 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 69 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 69 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 70 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 71 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 71 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 72 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 72 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 73 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 73 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 74 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 74 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 75 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 75 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 76 : Symbol not previously defined (_Div_16x16_S)
Error[152] DIGITAL THERMOMETERDT.ASM 76 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 77 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 77 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 78 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 78 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 79 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 79 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 80 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 80 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 82 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 83 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 83 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 84 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 85 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 85 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 86 : Symbol not previously defined (_Div_16x16_S)
Error[152] DIGITAL THERMOMETERDT.ASM 86 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 87 : Symbol not previously defined (R8)
Error[152] DIGITAL THERMOMETERDT.ASM 87 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 88 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 88 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 89 : Symbol not previously defined (R8)
Error[152] DIGITAL THERMOMETERDT.ASM 89 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 90 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 90 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 91 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 91 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 92 : Symbol not previously defined (_a)
Error[152] DIGITAL THERMOMETERDT.ASM 92 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 94 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 95 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 95 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 96 : Symbol not previously defined (_lcd)
Error[152] DIGITAL THERMOMETERDT.ASM 96 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 98 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 99 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 99 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 100 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 101 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 101 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 102 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 102 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 103 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 103 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 104 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 104 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 105 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 105 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 106 : Symbol not previously defined (_Div_16x16_S)
Error[152] DIGITAL THERMOMETERDT.ASM 106 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 107 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 107 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 108 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 108 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 109 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 109 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 110 : Symbol not previously defined (_t)
Error[152] DIGITAL THERMOMETERDT.ASM 110 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 112 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 113 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 113 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 114 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 115 : Symbol not previously defined (R4)
Error[152] DIGITAL THERMOMETERDT.ASM 115 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 116 : Symbol not previously defined (_Div_16x16_S)
Error[152] DIGITAL THERMOMETERDT.ASM 116 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 117 : Symbol not previously defined (R8)
Error[152] DIGITAL THERMOMETERDT.ASM 117 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 118 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 118 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 119 : Symbol not previously defined (R8)
Error[152] DIGITAL THERMOMETERDT.ASM 119 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 120 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 120 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 121 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 121 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 122 : Symbol not previously defined (_a)
Error[152] DIGITAL THERMOMETERDT.ASM 122 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 124 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 125 : Symbol not previously defined (R0)
Error[152] DIGITAL THERMOMETERDT.ASM 125 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 126 : Symbol not previously defined (_lcd)
Error[152] DIGITAL THERMOMETERDT.ASM 126 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 128 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 129 : Symbol not previously defined (FARG_Lcd_Out_row)
Error[152] DIGITAL THERMOMETERDT.ASM 129 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 130 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 131 : Symbol not previously defined (FARG_Lcd_Out_column)
Error[152] DIGITAL THERMOMETERDT.ASM 131 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 132 : Symbol not previously defined (_lcd)
Error[152] DIGITAL THERMOMETERDT.ASM 132 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 133 : Symbol not previously defined (FARG_Lcd_Out_text)
Error[152] DIGITAL THERMOMETERDT.ASM 133 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 134 : Symbol not previously defined (_Lcd_Out)
Error[152] DIGITAL THERMOMETERDT.ASM 134 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 136 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 137 : Symbol not previously defined (R11)
Error[152] DIGITAL THERMOMETERDT.ASM 137 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 138 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 139 : Symbol not previously defined (R12)
Error[152] DIGITAL THERMOMETERDT.ASM 139 : Executable code and data must be defined in an appropriate section
Warning[202] DIGITAL THERMOMETERDT.ASM 140 : Argument out of range. Least significant bits used.
Error[152] DIGITAL THERMOMETERDT.ASM 140 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 141 : Symbol not previously defined (R13)
Error[152] DIGITAL THERMOMETERDT.ASM 141 : Executable code and data must be defined in an appropriate section
Error[150] DIGITAL THERMOMETERDT.ASM 142 : Labels must be defined in a code or data section when making an object file
Error[113] DIGITAL THERMOMETERDT.ASM 143 : Symbol not previously defined (R13)
Error[152] DIGITAL THERMOMETERDT.ASM 143 : Executable code and data must be defined in an appropriate section
Error[151] DIGITAL THERMOMETERDT.ASM 144 : Operand contains unresolvable labels or is too complex
Error[152] DIGITAL THERMOMETERDT.ASM 144 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 145 : Symbol not previously defined (R12)
Error[152] DIGITAL THERMOMETERDT.ASM 145 : Executable code and data must be defined in an appropriate section
Error[151] DIGITAL THERMOMETERDT.ASM 146 : Operand contains unresolvable labels or is too complex
Error[152] DIGITAL THERMOMETERDT.ASM 146 : Executable code and data must be defined in an appropriate section
Error[113] DIGITAL THERMOMETERDT.ASM 147 : Symbol not previously defined (R11)
Error[152] DIGITAL THERMOMETERDT.ASM 147 : Executable code and data must be defined in an appropriate section
Error[151] DIGITAL THERMOMETERDT.ASM 148 : Operand contains unresolvable labels or is too complex
Error[152] DIGITAL THERMOMETERDT.ASM 148 : Executable code and data must be defined in an appropriate section
Error[152] DIGITAL THERMOMETERDT.ASM 149 : Executable code and data must be defined in an appropriate section
Error[151] DIGITAL THERMOMETERDT.ASM 151 : Operand contains unresolvable labels or is too complex
Error[152] DIGITAL THERMOMETERDT.ASM 151 : Executable code and data must be defined in an appropriate section
Error[150] DIGITAL THERMOMETERDT.ASM 153 : Labels must be defined in a code or data section when making an object file
Error[152] DIGITAL THERMOMETERDT.ASM 153 : Executable code and data must be defined in an appropriate section
Error[129] DIGITAL THERMOMETERDT.ASM 155 : Expected (END)
try the above code just by changing the microcontroller in project settings.. it will work..
thank u but i am beginner and i need the programme
Refer the datasheet of PIC 16F877, you need to change the register ADCON1.. that is the only modification..
please have u program of water regulator controlled with microcontroller pic 16F877
PIC Microcontroller Tutorials : http://www.electrosome.com/category/tutorials/pic-microcontroller-tutorials/
You can write these programs to PIC IC using PIC Programmer..
please tell me how to code these ics?
You can download codes and proteus files.. from above link…
i wanna its program code plz 🙂
http://www.electrosome.com/category/tutorials/pic-microcontroller-tutorials/
Read these tutorials, I think it may help you…. If you have any doubts after going through these tutorials, post your doubts in our newly developing forum http://www.electrosome.com/forum/
ou .. thankz ….
Rotate the stepper motor when the adc value read from LM35 become greater than a particular value…………
http://www.electrosome.com/stepper-motor-pic-microcontroller/
how i can make LM35 as the input of temperature sensor and stepper motor as the output. while the LM35 detect high temperature , the stepper motor will turn on??
The output of LM35 is connected to an analog pin of pic microcontroller………… and MCLR pin is connected to VDD….
why u dont use an analogique entry for PIC ??
MCLR/vpp/THV ? for what we use this pin !!
8mhz
what is the frequency for the chrystal?
Download Link : http://www.electrosome.com/wp-content/plugins/download-monitor/download.php?id=8
You Must login to download the file.
http://www.login.electrosome.com
Hello! where can I find the file? thanks alot! 🙂
Please Login and goto this link http://www.electrosome.com/wp-content/plugins/download-monitor/download.php?id=8
You can login at http://www.login.electrosome.com
How do I download the file? I have already registered but I still can’t download.
Thanks for the Share………..