Interfacing DHT11 Sensor with ESP8266
Contents
In this article, I am going to explain about interfacing of DHT11 sensor with ESP8266. For that we have to make only 3 connections between the DHT11 sensor and ESP8266. For the working demonstration we will be programing ESP8266 as a web server for displaying temperature and humidity in a web browser as per request.
Components Required
- ESP8266
- DHT11 Sensor
- USB Cable
- Connecting Wires
Hardware
Circuit Diagram
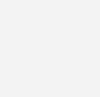
Connections
- DHT11 sensor (+) pin will be connected to 3.3V
- DHT11 sensor (-) pin will be connected to GND pin
- Output of DHT11 sensor is connected to GPIO5 pin of ESP8266.
software
Code
#include <ESP8266WiFi.h> #include "DHT.h" #define DHTTYPE DHT11 const char* ssid = "SERVER NAME"; const char* password = "SERVER PASSWORD"; WiFiServer server(80); const int DHTPin = 5; DHT dht(DHTPin, DHTTYPE); static char celsiusTemp[7]; static char fahrenheitTemp[7]; static char humidityTemp[7]; void setup() { Serial.begin(115200); delay(10); dht.begin(); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected"); server.begin(); Serial.println("Web server running. Waiting for the ESP IP..."); delay(10000); Serial.println(WiFi.localIP()); } void loop() { WiFiClient client = server.available(); if (client) { Serial.println("New client"); boolean blank_line = true; while (client.connected()) { if (client.available()) { char c = client.read(); if (c == '\n' && blank_line) { float h = dht.readHumidity(); float t = dht.readTemperature(); float f = dht.readTemperature(true); if (isnan(h) || isnan(t) || isnan(f)) { Serial.println("Failed to read from DHT sensor!"); strcpy(celsiusTemp,"Failed"); strcpy(fahrenheitTemp, "Failed"); strcpy(humidityTemp, "Failed"); } else { float hic = dht.computeHeatIndex(t, h, false); dtostrf(hic, 6, 2, celsiusTemp); float hif = dht.computeHeatIndex(f, h); dtostrf(hif, 6, 2, fahrenheitTemp); dtostrf(h, 6, 2, humidityTemp); } client.println("HTTP/1.1 200 OK"); client.println("Content-Type: text/html"); client.println("Connection: close"); client.println(); client.println("<!DOCTYPE HTML>"); client.println("<html>"); client.println("<head></head><body><h1>ESP8266 - Temperature and Humidity</h1><h3>Temperature in Celsius: "); client.println(celsiusTemp); client.println("*C</h3><h3>Temperature in Fahrenheit: "); client.println(fahrenheitTemp); client.println("*F</h3><h3>Humidity: "); client.println(humidityTemp); client.println("%</h3><h3>"); client.println("</body></html>"); break; } if (c == '\n') { blank_line = true; } else if (c != '\r') { blank_line = false; } } } delay(1); client.stop(); Serial.println("Client disconnected."); } }
Code Explanation:
We are including ESP8266 WiFi library which provides ESP8266 specific WiFi routines and we are calling it to connect to the network. Also we are including the library “DHT.h” which enables us to read temperature and humidity information from the sensor.
#include <ESP8266WiFi.h> #include "DHT.h"
Mention the type of the DHT sensor which we are using.
#define DHTTYPE DHT11
Get and enter the “ssid” and “password” i.e., your WiFi name and password.
const char* ssid = "SERVER NAME"; const char* password = "SERVER PASSWORD";
Declare the Web Server on port 80. Port 80 is the default port, that a web server “listens to” or expects to receive from a web client.
WiFiServer server(80);
DHTPin is a constant integer type, constants won’t change throughout the program. It is set to pin number 5 (GPIO05) of ESP8266 and initialize DHT sensor.
const int DHTPin = 5; DHT dht(DHTPin, DHTTYPE);
Declare the temporary variables with the type of static char for temperature and humidity.
static char celsiusTemp[7]; static char fahrenheitTemp[7]; static char humidityTemp[7];
Put your setup or configuration code in the setup function, it will only run once during the startup.
Here in the setup function, it will connect to WiFi Network and also initialize serial communication for debugging and logging with a baud rate of 115200.
void setup() { Serial.begin(115200); delay(10); dht.begin();
Actual connection to WiFi is initialized by calling below instructions.
Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, password);
Connection process can take couple of seconds. While() loop checks the module connection with the WiFi network. If it is connected to WiFi then it will display WiFi connected message on the serial monitor, otherwise it will continue to display “dots (……)”.
while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected");
It is printing the IP address of our ESP8266 module. This IP address is given by the DHCP server running on the WiFi router. For accessing it, we need to connect our system to the same WiFi network (our system will get another IP address from the router DHCP server) and we need to enter the IP address of the ESP8266 in the browser of our system.
server.begin(); Serial.println("Web server running. Waiting for the ESP IP..."); delay(10000); Serial.println(WiFi.localIP()); }
Put your main code in void loop() function to run repeatedly.
Waiting and checking for a client to connect.
Note : Client means browser. When we are loading ESP8266 IP address in a browser it is actually sending a HTTP request to the ESP8266 web server listening on the TCP port 80.
void loop() { WiFiClient client = server.available();
If new client available, the boolean variable “client” will be true and we proceed to process the request.
if (client) { Serial.println("New client"); boolean blank_line = true; while (client.connected()) { if (client.available()) { char c = client.read();
If client available then read humidity, temperature in Celsius (the default) and Fahrenheit (isFahrenheit = true) .
if (c == '\n' && blank_line) { float h = dht.readHumidity(); float t = dht.readTemperature(); float f = dht.readTemperature(true);
Check if any reading failed and exit early. If reading failed then we will send “Failed to read from DHT sensor!” in the HTTP response.
if (isnan(h) || isnan(t) || isnan(f)) { Serial.println("Failed to read from DHT sensor!"); strcpy(celsiusTemp,"Failed"); strcpy(fahrenheitTemp, "Failed"); strcpy(humidityTemp, "Failed"); }
If it reads successfully, then computes temperature values in Celsius + Fahrenheit and Humidity.
else { float hic = dht.computeHeatIndex(t, h, false); dtostrf(hic, 6, 2, celsiusTemp); float hif = dht.computeHeatIndex(f, h); dtostrf(hif, 6, 2, fahrenheitTemp); dtostrf(h, 6, 2, humidityTemp); }
HTML code to create a web page which displays the temperature and humidity value.
client.println("HTTP/1.1 200 OK"); client.println("Content-Type: text/html"); client.println("Connection: close"); client.println(); client.println("<!DOCTYPE HTML>"); client.println("<html>"); client.println("<head></head><body><h1>ESP8266 - Temperature and Humidity</h1><h3>Temperature in Celsius: "); client.println(celsiusTemp); client.println("*C</h3><h3>Temperature in Fahrenheit: "); client.println(fahrenheitTemp); client.println("*F</h3><h3>Humidity: "); client.println(humidityTemp); client.println("%</h3><h3>"); client.println("</body></html>"); break; } if (c == '\n') { blank_line = true; } else if (c != '\r') { blank_line = false; } } } delay(1); client.stop(); Serial.println("Client disconnected."); } }
Practical Implementation
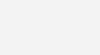
Working
In this project we are using DHT11 sensor to monitor the temperature and humidity of the place where it is located. The heart of the is project is ESP8266 WiFi SoC. ESP8266 is programmed as a web server running on default HTTP port 80. When it receives a request from a client (web browser), it will read data from the DHT11 sensor and send the response to be displayed in the browser.
How to monitor data over Internet ?
We are not going to explain this in detail in this tutorial. Most of the wifi routers available in the market supports DDNS (Dynamic DNS) and port forwarding. This can be used to access the web page served by ESP8266 over internet. In real IoT applications we need to connect ESP8266 to a internet server. We will explain those in next tutorials.
How can you add a trigger to send email. For example if the temperature reaches 75 or 75 Degrees Fahrenheit to send an email warning the temperature is increasing.