Digital Thermometer using Arduino and DS18B20 Sensor
Contents
In this project, we are going to make a Digital Thermometer using Arduino Uno. We will use DS18B20 temperature sensor to sense the temperature and Nokia 5110 LCD to display it. DS18B20 is a 1-Wire digital temperature sensor manufactured by Maxim Integrated and is capable of reporting degree celsius with 9 ~ 12 bit precision.
Components Required
- Arduino Uno
- DS18B20 Temperature Sensor
- Nokia 5110 LCD
- 1KΩ Potentiometer
- 10KΩ Resistors – 4
- 4.7KΩ Resistor
- 1KΩ Resistor
- 330Ω Resistor
- Connecting Wires
- Breadboard
Circuit Diagram
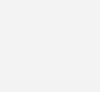
Explanation
First we will connect Nokia 5110 LCD to Arduino as follows.
- Connect the GND pin of Nokia 5110 LCD to GND of Arduino.
- Connect the LED pin of Nokia 5110 LCD to middle pin of 1KΩ potentiometer through 330Ω resistor and connect other two pins of the potentiometer to VCC, GND.
- Connect the VCC pin of Nokia 5110 LCD to 3.3V output pin of Arduino.
- Connect the CLK pin of Nokia 5110 LCD to Pin 8 of Arduino through a 10KΩ resistor.
- Connect the DIN pin of Nokia 5110 LCD to Pin 9 of Arduino through a 10KΩ resistor.
- Connect the D/C pin of Nokia 5110 LCD to Pin 10 of Arduino through a 10KΩ resistor.
- Connect the SCE pin of Nokia 5110 LCD to Pin 11 of Arduino through a 1KΩ resistor.
- Connect the RST pin of Nokia 5110 LCD to Pin 12 of Arduino through a 10KΩ resistor.
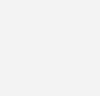
Then connect DS18B20 temperature sensor to Arduino as follows.
- Connect left pin of DS18B20 to GND of Arduino.
- Connect right pin of DS18B20 to 5V output of Arduino.
- Connect middle pin of DS18B20 to Pin 2Â of Arduino
- Connect a 4.7KΩ resistor from middle pin of DS18B20 to 5V as pull up.
Program
Libraries
You need to manually add following libraries to Arduino IDE as it is not included by default. You can ignore it if you already added it. Otherwise you can do following steps for that.
- Download Libraries
- Open Arduino IDE
- Go to Sketch >> Include Library >> Add .ZIP Library
- Select the downloaded ZIP file and press open
Code
#include <OneWire.h> #include <DallasTemperature.h> #include <LCD5110_Graph.h> #define ONE_WIRE_BUS 2 LCD5110 Nokia_5110_LCD(8,9,10,12,11); OneWire oneWire(ONE_WIRE_BUS); DallasTemperature ds18b20(&oneWire); extern unsigned char BigNumbers[]; char temperature[6]; float temp = 0; const uint8_t logo[] PROGMEM={ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x3C, 0xC0, 0x30, 0x0C, 0x03, 0x0C, 0x30, 0xC0, 0x3C, 0x03, 0x00, 0xFF, 0x89, 0x89, 0x89, 0x00, 0x00, 0xFF, 0x80, 0x80, 0x80, 0x00, 0x7E, 0x81, 0x81, 0x81, 0x42, 0x00, 0x7E, 0x81, 0x81, 0x81, 0x81, 0x7E, 0x00, 0x00, 0xFF, 0x03, 0x3C, 0xC0, 0xC0, 0x3C, 0x03, 0xFF, 0x00, 0x00, 0xFF, 0x89, 0x89, 0x89, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x40, 0x40, 0xC0, 0x40, 0x40, 0x00, 0x80, 0x40, 0x40, 0x40, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x07, 0x08, 0x08, 0x08, 0x07, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFE, 0x12, 0x12, 0x12, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0x00, 0xFE, 0x12, 0x12, 0x12, 0x00, 0x00, 0xFC, 0x02, 0x02, 0x02, 0x84, 0x02, 0x02, 0xFE, 0x02, 0x02, 0x00, 0x00, 0xFE, 0x22, 0x22, 0x62, 0x9C, 0x00, 0x00, 0xFC, 0x02, 0x02, 0x02, 0x02, 0xFC, 0x00, 0x00, 0x8C, 0x12, 0x22, 0xC4, 0x00, 0x00, 0xFC, 0x02, 0x02, 0x02, 0x02, 0xFC, 0x00, 0x00, 0xFE, 0x06, 0x78, 0x80, 0x80, 0x78, 0x06, 0xFE, 0x00, 0x00, 0xFE, 0x12, 0x12, 0x12, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x01, 0x01, 0x01, 0x00, 0x00, 0x01, 0x01, 0x01, 0x01, 0x00, 0x01, 0x01, 0x01, 0x01, 0x00, 0x00, 0x00, 0x01, 0x01, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0x01, 0x01, 0x01, 0x00, 0x00, 0x00, 0x00, 0x01, 0x01, 0x00, 0x00, 0x00, 0x00, 0x01, 0x01, 0x01, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x01, 0x01, 0x00, 0x00, 0x01, 0x00, 0x00, 0x01, 0x01, 0x01, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, }; const uint8_t thermometer_sign[] PROGMEM={ 0x00, 0x00, 0x00, 0x00, 0x00, 0xFC, 0xFE, 0xFE, 0x1F, 0x0F, 0x0F, 0x0F, 0x3F, 0xFE, 0xFE, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xFF, 0xC0, 0xC0, 0xC0, 0xC0, 0xC0, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xC0, 0xE0, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE0, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7C, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x07, 0x0F, 0x1F, 0x1F, 0x3F, 0x3F, 0x3F, 0x3F, 0x3F, 0x3F, 0x1F, 0x1F, 0x1F, 0x0F, 0x07, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, }; void setup() { Nokia_5110_LCD.InitLCD(); Nokia_5110_LCD.setFont(BigNumbers); ds18b20.begin(); Nokia_5110_LCD.drawBitmap( 0, 0, logo, 84, 48); Nokia_5110_LCD.update(); delay(3000); } void loop() { Nokia_5110_LCD.clrScr(); Nokia_5110_LCD.drawBitmap( 0, 0, thermometer_sign, 84, 48); ds18b20.requestTemperatures(); temp = ds18b20.getTempCByIndex(0); dtostrf(temp, 3, 1, temperature); Nokia_5110_LCD.print(temperature,25,11); Nokia_5110_LCD.update(); delay(1000); }
Explanation
Firstly, we are adding libraries for getting temperature data from DS18B20 temperature sensor and for displaying data in Nokia 5110 LCD. Dalla’s DS18B20 is using 1-Wire protocol, so OneWire library is used for its communication. DallasTemperature library will read data from DS18B20 and return the current temperature in celsius.
#include <OneWire.h> #include <DallasTemperature.h> #include <LCD5110_Graph.h>
Next we are defining pins where we have connected Nokia 5110 LCD and DS18B20 temperature sensor.
#define ONE_WIRE_BUS 2 LCD5110 Nokia_5110_LCD(8,9,10,12,11); OneWire oneWire(ONE_WIRE_BUS); DallasTemperature ds18b20(&oneWire);
Then we initialize two arrays in the program memory. The first array (logo) is for displaying “WELCOME TO ELECTROSOME” and second array (thermometer_sign) is for displaying thermometer symbol in the display.
const uint8_t logo[] PROGMEM={ 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
In the setup function, we are doing following tasks.
- Initialising the LCD
- Setting the font for displaying temperature values
- Initializing Arduino for reading data from DS18B20
- Displaying “WELCOME TO ELECTROSOME”
Nokia_5110_LCD.InitLCD(); Nokia_5110_LCD.setFont(BigNumbers); ds18b20.begin(); Nokia_5110_LCD.drawBitmap( 0, 0, logo, 84, 48); Nokia_5110_LCD.update();
Then in the loop we are clearing the LCD screen and displaying the thermometer logo in the display.
Nokia_5110_LCD.clrScr(); Nokia_5110_LCD.drawBitmap( 0, 0, thermometer_sign, 84, 48);
After this, we are reading the temperature from DS18B20 sensor and storing it to the variable “temp”. Then we are displaying it in the LCD.
ds18b20.requestTemperatures(); temp = ds18b20.getTempCByIndex(0); dtostrf(temp, 3, 1, temperature); Nokia_5110_LCD.print(temperature,25,11); Nokia_5110_LCD.update();
Working
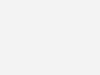
As we explained above DS18B20 is a digital temperature sensor which uses 1-Wire bus for communication. This means that we need to use only one digital pin of Arduino (ground is common) for reading temperature from it. We are using OneWire library and DallasTemperature library for this purpose.
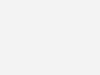
We are displaying 2 static data in the LCD, one is welcome text “WELCOME TO ELECTROSOME” and another one is thermometer logo as shown above. So we are defining bitmap arrays (logo and thermometer_sign) in the program for this. Temperature value displayed in the LCD is a dynamic data which we will read from the temperature sensor. You can see the video of this project below.